c++11 获取类成员中的属性并以字符串方式输出
时间: 2024-05-05 17:22:14 浏览: 20
你可以使用反射技术来获取类成员中的属性并以字符串方式输出。反射是一种在运行时检查、访问和修改程序元素的能力。在C++11中,可以使用一些库来实现反射,例如Boost.Reflection和RTTR(Run Time Type Reflection)。
以下是一个使用Boost.Reflection库的示例代码,可以获取类成员中的属性并以字符串方式输出:
```c++
#include <iostream>
#include <boost/reflection.hpp>
class MyClass {
public:
MyClass(int id, const std::string& name) : id_(id), name_(name) {}
int get_id() const { return id_; }
const std::string& get_name() const { return name_; }
private:
int id_;
std::string name_;
};
int main() {
MyClass obj(1, "Alice");
boost::reflection::Object obj_ref(obj);
boost::reflection::Type obj_type = obj_ref.GetType();
std::vector<boost::reflection::Property> properties = obj_type.GetProperties();
for (const auto& property : properties) {
std::cout << property.GetName() << ": " << property.GetValue(obj_ref) << std::endl;
}
return 0;
}
```
在上面的示例代码中,我们定义了一个名为MyClass的类,并在其中定义了成员变量id_和name_,以及成员函数get_id()和get_name()。接下来,在main函数中,我们创建了一个MyClass对象,并使用boost::reflection::Object类来获取对象的引用。然后,我们使用boost::reflection::Type类来获取对象的类型,并使用GetProperties()函数获取对象的所有属性。最后,我们使用属性的名称和GetValue()函数来输出属性的值。
需要注意的是,Boost.Reflection是一个第三方库,需要在项目中添加相应的头文件和链接库。如果不想使用第三方库,也可以手动实现反射功能。
相关推荐
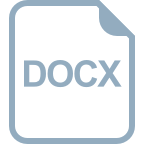
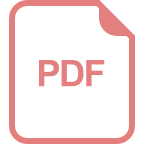
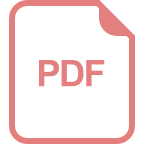












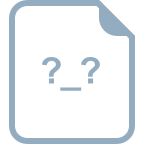
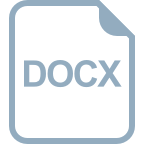
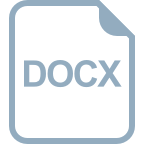