python如何多线程频繁高效率查询sqlite3数据库是否存在要查询的内容
时间: 2023-05-26 07:03:09 浏览: 291
可以使用Python内置的threading模块来实现多线程,同时使用sqlite3模块来操作数据库。
以下是一个示例代码:
```python
import threading
import sqlite3
# 创建一个SQLite3连接
conn = sqlite3.connect('test.db')
# 创建一个游标
cursor = conn.cursor()
# 定义要查询的内容
query_content = 'hello'
# 定义一个线程类
class SearchThread(threading.Thread):
def __init__(self, query):
threading.Thread.__init__(self)
self.query = query
def run(self):
# 查询是否存在要查询的内容并输出结果
cursor.execute("SELECT * FROM test WHERE content=?", (self.query,))
result = cursor.fetchone()
if result:
print(self.query, 'exists')
else:
print(self.query, 'not exists')
# 创建5个线程来查询要查询的内容
for i in range(5):
t = SearchThread(query_content)
t.start()
# 关闭游标和连接
cursor.close()
conn.close()
```
以上代码中,首先创建了一个SQLite3连接和游标。然后定义了要查询的内容为"hello"。接着定义了一个线程类SearchThread,继承自threading.Thread,并在run方法中查询是否存在要查询的内容,并输出结果。最后创建了5个SearchThread线程来查询要查询的内容。
可以看到,这个示例代码中,多个线程可以同时查询SQLite3数据库,提高了查询效率。
阅读全文
相关推荐
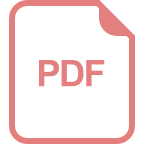
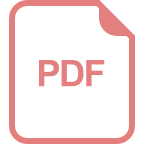
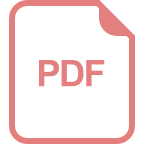
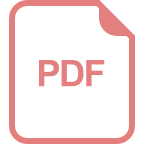
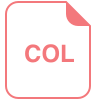
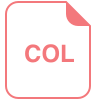
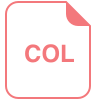
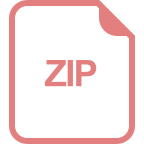
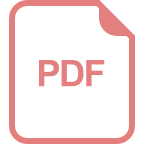
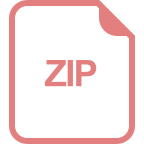
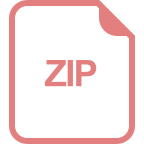
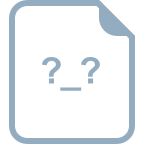
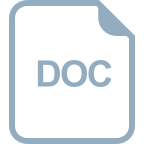
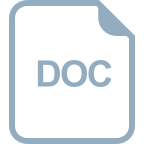
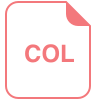
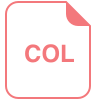
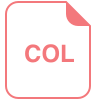
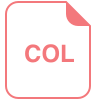
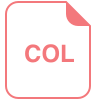