在linux下编写一个can通信接收程序,要求接收两个不同id的数据帧,并使用多线程,使得接收线程不影响主线程
时间: 2024-03-01 20:56:14 浏览: 192
要在Linux下编写一个CAN通信接收程序,您需要使用CAN接口库(SocketCAN或can-utils)和编程语言(例如C或Python)。以下是一个使用C编写的CAN通信接收程序示例,该程序可以接收两个不同ID的数据帧,并使用多线程来避免接收线程阻塞主线程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <errno.h>
#include <pthread.h>
#include <sys/socket.h>
#include <sys/ioctl.h>
#include <net/if.h>
#include <linux/can.h>
#include <linux/can/raw.h>
#define CAN_INTERFACE "can0" // CAN接口名称
#define CAN_ID1 0x123 // 第一个接收的CAN ID
#define CAN_ID2 0x456 // 第二个接收的CAN ID
void *can_receive_function(void *arg) {
int s;
struct sockaddr_can addr;
struct ifreq ifr;
struct can_frame frame;
int nbytes;
// 创建CAN套接字
if ((s = socket(PF_CAN, SOCK_RAW, CAN_RAW)) < 0) {
perror("socket");
exit(EXIT_FAILURE);
}
// 获取CAN接口信息
strcpy(ifr.ifr_name, CAN_INTERFACE);
ioctl(s, SIOCGIFINDEX, &ifr);
// 绑定CAN套接字到CAN接口
addr.can_family = AF_CAN;
addr.can_ifindex = ifr.ifr_ifindex;
if (bind(s, (struct sockaddr *)&addr, sizeof(addr)) < 0) {
perror("bind");
exit(EXIT_FAILURE);
}
// 接收CAN数据帧
while (1) {
nbytes = read(s, &frame, sizeof(struct can_frame));
if (nbytes < 0) {
perror("read");
break;
}
// 根据CAN ID判断是否为需要接收的数据帧
if (frame.can_id == CAN_ID1) {
printf("Received CAN frame with ID 0x%x\n", frame.can_id);
// 处理第一个接收的数据帧
} else if (frame.can_id == CAN_ID2) {
printf("Received CAN frame with ID 0x%x\n", frame.can_id);
// 处理第二个接收的数据帧
}
}
// 关闭CAN套接字
close(s);
return NULL;
}
int main() {
pthread_t can_receive_thread;
// 创建CAN接收线程
if (pthread_create(&can_receive_thread, NULL, can_receive_function, NULL) != 0) {
perror("pthread_create");
exit(EXIT_FAILURE);
}
// 主线程继续执行其他任务
while (1) {
// 可以在这里执行其他任务,不受接收线程影响
sleep(1);
}
return 0;
}
```
在上面的示例中,主线程在创建CAN接收线程后继续执行其他任务,而CAN接收线程在不断地接收CAN数据帧并进行处理,不会影响主线程的执行。
阅读全文
相关推荐
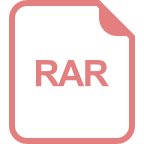














