EF Core写一个根据日期查询员工的上下班打卡记录
时间: 2024-01-20 20:04:29 浏览: 180
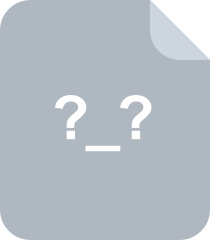
员工打卡记录
假设你已经有了一个 `Employee` 实体类和一个 `Attendance` 实体类,其中 `Attendance` 记录了员工的上下班打卡记录,包括打卡日期、上班打卡时间和下班打卡时间等信息。你需要查询某个员工在指定日期的上下班打卡记录,可以按照以下步骤进行操作:
1. 创建一个 `DbContext` 类,用于管理实体类和数据库连接。
```csharp
public class MyDbContext : DbContext
{
public DbSet<Employee> Employees { get; set; }
public DbSet<Attendance> Attendances { get; set; }
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
// 配置数据库连接字符串
optionsBuilder.UseSqlServer("Server=localhost;Database=mydb;User Id=myuser;Password=mypassword;");
}
}
```
2. 在 `Attendance` 实体类中定义一个 `Employee` 属性,用于关联员工实体。
```csharp
public class Attendance
{
public int Id { get; set; }
public DateTime Date { get; set; }
public DateTime? ClockIn { get; set; }
public DateTime? ClockOut { get; set; }
public int EmployeeId { get; set; }
public Employee Employee { get; set; }
}
```
3. 在 `Employee` 实体类中定义一个 `Attendances` 属性,用于关联打卡记录实体集合。
```csharp
public class Employee
{
public int Id { get; set; }
public string Name { get; set; }
public ICollection<Attendance> Attendances { get; set; }
}
```
4. 编写查询代码。可以使用 LINQ 表达式查询指定日期的员工打卡记录,示例代码如下:
```csharp
using (var db = new MyDbContext())
{
var employeeId = 1; // 员工 ID
var date = DateTime.Parse("2022-01-01"); // 打卡日期
var query = from a in db.Attendances
where a.EmployeeId == employeeId && a.Date == date
select a;
var results = query.ToList();
foreach (var result in results)
{
Console.WriteLine($"{result.ClockIn} - {result.ClockOut}");
}
}
```
上述代码中,首先创建了一个 `MyDbContext` 实例,然后使用 LINQ 表达式查询指定员工和日期的打卡记录,最后将结果输出到控制台。需要注意的是,查询结果是一个 `List<Attendance>` 集合,其中包含了员工在指定日期的所有打卡记录。如果某个打卡时间为空,对应的属性值将会是 `null`。
阅读全文
相关推荐
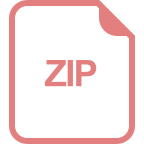
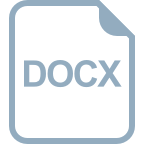


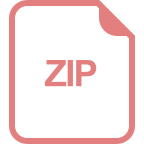
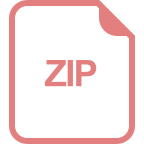
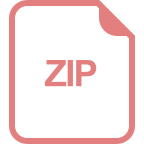
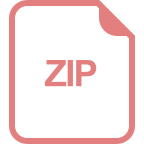
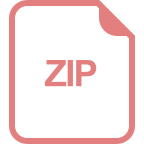
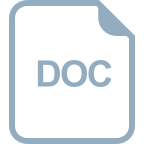
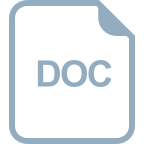
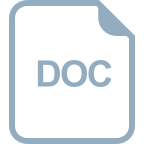
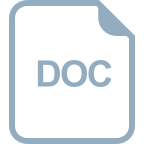



