java读取excel的数据
时间: 2023-10-26 19:06:18 浏览: 84
可以使用Apache POI库来读取Excel文件的数据,具体步骤可以参考以下示例代码:
```
// 导入POI库的相关类
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
// 读取Excel文件中的数据
try {
// 加载Excel文件
File file = new File("path/to/excel/file.xlsx");
Workbook workbook = null;
if (file.exists()) {
FileInputStream fis = new FileInputStream(file);
if (file.getName().endsWith(".xls")) {
workbook = new HSSFWorkbook(fis); // Excel 2003的格式,使用HSSFWorkbook
} else if (file.getName().endsWith(".xlsx")) {
workbook = new XSSFWorkbook(fis); // Excel 2007及以上的格式,使用XSSFWorkbook
}
fis.close();
}
if (workbook == null) {
System.err.println("文件不存在或格式不正确!");
return;
}
// 读取Excel中的数据
Sheet sheet = workbook.getSheetAt(0); // 获取第一个工作表
int rowCount = sheet.getPhysicalNumberOfRows(); // 获取行数
for (int i = 0; i < rowCount; i++) {
Row row = sheet.getRow(i); // 获取一行数据
if (row == null) {
continue;
}
int cellCount = row.getPhysicalNumberOfCells(); // 获取列数
for (int j = 0; j < cellCount; j++) {
Cell cell = row.getCell(j); // 获取单元格
if (cell == null) {
continue;
}
// 处理单元格数据,如:
switch(cell.getCellType()) {
case BOOLEAN:
System.out.println(cell.getBooleanCellValue());
break;
case NUMERIC:
System.out.println(cell.getNumericCellValue());
break;
case STRING:
System.out.println(cell.getStringCellValue());
break;
default:
System.out.println(cell.toString());
break;
}
}
}
// 关闭Excel文件
workbook.close();
} catch (IOException e) {
e.printStackTrace();
}
```
以上代码使用Apache POI库读取Excel文件中的数据,并输出到控制台。具体可以根据需要自行修改,例如输出到文件或者数据库等。
阅读全文
相关推荐
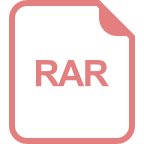
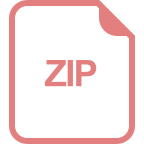















