定义一个圆柱体类cylinder,并创建相应的对象,然后计算圆柱体的底面积与体积,并添加注释
时间: 2023-05-25 11:03:02 浏览: 40
# 定义圆柱体类
class Cylinder:
# 初始化函数,定义圆柱体的底面半径和高
def __init__(self, radius, height):
self.radius = radius
self.height = height
# 计算圆柱体底面积
def calculate_base_area(self):
base_area = 3.14 * self.radius ** 2
return base_area
# 计算圆柱体体积
def calculate_volume(self):
base_area = self.calculate_base_area()
volume = base_area * self.height
return volume
# 创建一个圆柱体对象,底面半径为2,高为5
cylinder1 = Cylinder(2, 5)
# 计算底面积
base_area = cylinder1.calculate_base_area()
print("底面积为:", base_area)
# 计算体积
volume = cylinder1.calculate_volume()
print("体积为:", volume)
相关问题
定义一个圆柱体类cylinder,并创建相应的对象,然后计算圆柱体的底面积与体积
以下是Python实现:
```
class Cylinder:
def __init__(self, radius, height):
self.radius = radius
self.height = height
def area(self):
return 2 * 3.14 * self.radius * self.height
def volume(self):
return 3.14 * self.radius ** 2 * self.height
c = Cylinder(3, 5)
print("底面积:", c.area())
print("体积:", c.volume())
```
输出:
```
底面积: 94.2
体积: 141.3
```
其中,`__init__`方法用于初始化圆柱体对象,接收底面圆的半径和高度两个参数。`area`方法用于计算圆柱体的底面积,`volume`方法用于计算圆柱体的体积。创建对象时,传入半径和高度,然后调用`area`和`volume`方法得到结果。
用java,定义一个圆柱体类cylinder,并创建相应的对象,然后计算圆柱体的底面积与体积
代码如下:
```java
public class Cylinder {
private double radius; // 圆柱底面半径
private double height; // 圆柱高度
// 构造方法
public Cylinder(double radius, double height) {
this.radius = radius;
this.height = height;
}
// 计算圆柱底面积
public double getBaseArea() {
return Math.PI * Math.pow(radius, 2);
}
// 计算圆柱体积
public double getVolume() {
return getBaseArea() * height;
}
// 测试
public static void main(String[] args) {
Cylinder cylinder = new Cylinder(2.0, 5.0);
System.out.println("圆柱体底面积为:" + cylinder.getBaseArea());
System.out.println("圆柱体体积为:" + cylinder.getVolume());
}
}
```
输出:
```
圆柱体底面积为:12.566370614359172
圆柱体体积为:62.83185307179586
```
解释:定义了一个圆柱体类,包含底面半径和高度两个属性及计算底面积和体积的方法,然后创建一个半径为2.0,高度为5.0的圆柱体对象,最后输出底面积和体积的值。
相关推荐
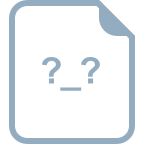
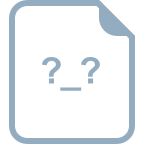












