new Promise()
时间: 2023-07-21 21:02:56 浏览: 67
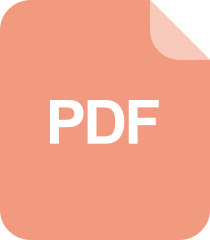
Vue ElementUi同时校验多个表单(巧用new promise)

Promise是JavaScript中的一个内置对象,用于处理异步操作。它表示一个异步操作的最终完成或失败,并可以在操作完成后返回结果或错误信息。
在创建Promise对象时,我们需要传入一个执行器函数作为参数,该函数有两个参数:resolve和reject。resolve用于将Promise的状态从pending(进行中)改为fulfilled(已完成),并返回一个结果值;reject用于将Promise的状态从pending改为rejected(已失败),并返回一个错误信息。
下面是一个使用Promise的示例:
```javascript
const promise = new Promise((resolve, reject) => {
// 异步操作,例如发送请求或读取文件等
// 如果操作成功,调用resolve并传入结果值
// 如果操作失败,调用reject并传入错误信息
});
```
我们可以通过使用then方法来处理Promise对象的结果:
```javascript
promise.then(result => {
// 处理操作成功的结果
}).catch(error => {
// 处理操作失败的错误信息
});
```
需要注意的是,Promise可以链式调用多个then方法来处理多个异步操作,每个then方法都会返回一个新的Promise对象。
阅读全文
相关推荐
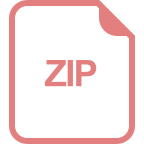
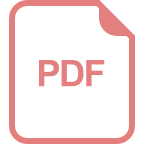















