C语言用栈求解迷宫问题的所有路经和最短路径
时间: 2023-05-20 13:02:52 浏览: 110
对于迷宫问题,可以使用深度优先搜索算法来求解所有路径,也可以使用广度优先搜索算法来求解最短路径。使用栈可以实现深度优先搜索算法,以下是C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_ROW 10
#define MAX_COL 10
int maze[MAX_ROW][MAX_COL] = {
{0, 1, 0, 0, 0, 1, 0, 1, 0, 0},
{0, 0, 0, 1, 0, 0, 0, 1, 0, 1},
{0, 1, 0, 1, 0, 1, 0, 0, 0, 0},
{0, 1, 0, 0, 0, 1, 0, 1, 1, 0},
{0, 1, 1, 1, 0, 0, 0, 0, 1, 0},
{0, 0, 0, 0, 0, 1, 0, 1, 0, 0},
{0, 1, 1, 1, 0, 0, 0, 1, 1, 0},
{0, 1, 0, 0, 0, 1, 0, 0, 0, 0},
{0, 1, 1, 1, 0, 0, 0, 1, 1, 1},
{0, 0, 0, 0, 0, 1, 0, 0, 0, 0}
};
typedef struct {
int row, col;
} Position;
Position stack[MAX_ROW * MAX_COL];
int top = -1;
void push(Position p) {
stack[++top] = p;
}
Position pop() {
return stack[top--];
}
int is_empty() {
return top == -1;
}
int maze_path(int maze[][MAX_COL], int start_row, int start_col, int end_row, int end_col) {
Position cur = {start_row, start_col};
maze[cur.row][cur.col] = 2;
push(cur);
while (!is_empty()) {
cur = pop();
if (cur.row == end_row && cur.col == end_col) {
printf("(%d, %d)", cur.row, cur.col);
return 1;
}
if (cur.col + 1 < MAX_COL && maze[cur.row][cur.col + 1] == 0) {
maze[cur.row][cur.col + 1] = 2;
push((Position){cur.row, cur.col + 1});
}
if (cur.row + 1 < MAX_ROW && maze[cur.row + 1][cur.col] == 0) {
maze[cur.row + 1][cur.col] = 2;
push((Position){cur.row + 1, cur.col});
}
if (cur.col - 1 >= 0 && maze[cur.row][cur.col - 1] == 0) {
maze[cur.row][cur.col - 1] = 2;
push((Position){cur.row, cur.col - 1});
}
if (cur.row - 1 >= 0 && maze[cur.row - 1][cur.col] == 0) {
maze[cur.row - 1][cur.col] = 2;
push((Position){cur.row - 1, cur.col});
}
}
return 0;
}
int main() {
int start_row = 0, start_col = 0;
int end_row = 9, end_col = 9;
if (maze_path(maze, start_row, start_col, end_row, end_col)) {
printf("\n");
} else {
printf("No path found.\n");
}
return 0;
}
```
以上代码可以求解迷宫问题的一条路径,如果要求解所有路径,可以在找到一条路径后,将该路径上的所有点都标记为已访问,然后继续搜索,直到所有路径都被找到为止。
阅读全文
相关推荐
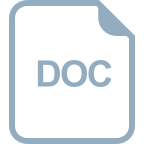
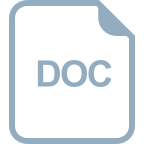
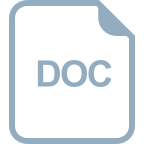

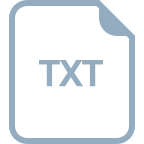

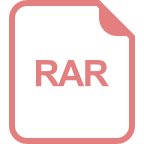
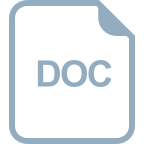
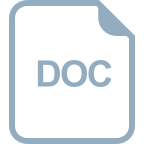
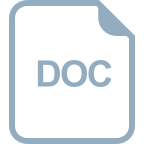
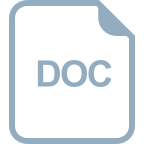
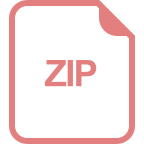
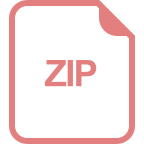
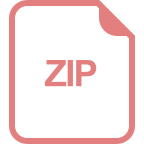
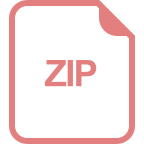