python已经用了pgp私钥产生签名,怎么使用公钥解密
时间: 2024-03-27 07:37:45 浏览: 72
公钥不能用于解密,只能用于验证签名的真实性。如果您想要解密数据,需要使用私钥来进行解密。如果您已经使用私钥进行签名,则可以使用公钥来验证签名的真实性。具体的操作步骤如下:
1. 首先,需要将公钥与私钥对应起来。如果您已经有了私钥,则可以使用以下命令生成公钥:
```
openssl rsa -in private.key -pubout -out public.key
```
其中,private.key 是您的私钥文件名,public.key 是您要生成的公钥文件名。
2. 然后,需要使用公钥来验证签名的真实性。具体的操作步骤如下:
首先,需要将签名与原始数据保存到文件中。假设签名保存在 signature.txt 文件中,原始数据保存在 data.txt 文件中。
```
openssl dgst -sha256 -verify public.key -signature signature.txt data.txt
```
其中,public.key 是您的公钥文件名,signature.txt 是签名文件名,data.txt 是原始数据文件名。
如果验证成功,则会输出 Verified OK 信息。如果验证失败,则会输出 Verification Failure 信息。
请注意,如果您的数据是使用非对称加密算法加密的,则需要使用相应的私钥进行解密。如果您的数据是使用对称加密算法加密的,则需要使用相应的密钥进行解密。
相关问题
如何用Python语言实现PGP
PGP(Pretty Good Privacy)是一种加密和数字签名程序。它使用公钥密码学来提供安全的通信和数据存储。在Python中,我们可以使用PyCrypto库来实现PGP。
以下是一个简单的示例,演示如何使用Python实现PGP:
```python
from Crypto.PublicKey import RSA
from Crypto.Cipher import PKCS1_OAEP
from Crypto.Signature import pkcs1_15
from Crypto.Hash import SHA256
# 生成一个新的RSA密钥对
key = RSA.generate(2048)
# 保存私钥到文件
with open('private.pem', 'wb') as f:
f.write(key.export_key())
# 保存公钥到文件
with open('public.pem', 'wb') as f:
f.write(key.publickey().export_key())
# 加载私钥
with open('private.pem', 'rb') as f:
private_key = RSA.import_key(f.read())
# 加载公钥
with open('public.pem', 'rb') as f:
public_key = RSA.import_key(f.read())
# 加密和解密
cipher = PKCS1_OAEP.new(public_key)
encrypted_data = cipher.encrypt(b'Hello World')
print(encrypted_data)
cipher = PKCS1_OAEP.new(private_key)
decrypted_data = cipher.decrypt(encrypted_data)
print(decrypted_data)
# 数字签名和验证
message = b'Hello World'
hash = SHA256.new(message)
signature = pkcs1_15.new(private_key).sign(hash)
print(signature)
try:
pkcs1_15.new(public_key).verify(hash, signature)
print('Signature is valid')
except (ValueError, TypeError):
print('Signature is invalid')
```
这个示例展示了如何生成一个新的RSA密钥对,如何加载私钥和公钥,如何使用公钥加密和私钥解密数据,以及如何使用私钥进行数字签名和使用公钥进行验证。请注意,这只是一个简单的示例,实际应用中可能需要更多的安全措施和代码优化。
PGP模拟公钥加解密
PGP(Pretty Good Privacy)是一种加密通信协议,用于保护数据的机密性和完整性。在PGP中,公钥加密算法用于加密和解密数据。
以下是一个简单的PGP模拟公钥加解密的Python代码示例:
```python
from Crypto.PublicKey import RSA
from Crypto.Cipher import PKCS1_OAEP
# 生成公钥和私钥
key = RSA.generate(2048)
private_key = key.export_key()
public_key = key.publickey().export_key()
# 加密函数
def encrypt(public_key, plaintext):
key = RSA.import_key(public_key)
cipher_rsa = PKCS1_OAEP.new(key)
ciphertext = cipher_rsa.encrypt(plaintext)
return ciphertext
# 解密函数
def decrypt(private_key, ciphertext):
key = RSA.import_key(private_key)
cipher_rsa = PKCS1_OAEP.new(key)
plaintext = cipher_rsa.decrypt(ciphertext)
return plaintext
# 测试加解密
plaintext = b'Hello, world!'
ciphertext = encrypt(public_key, plaintext)
decrypted = decrypt(private_key, ciphertext)
print(f"Plaintext: {plaintext}")
print(f"Ciphertext: {ciphertext}")
print(f"Decrypted: {decrypted}")
```
在这个例子中,我们使用了Python加密库`pycryptodome`中的RSA加密算法和PKCS#1 OAEP填充方式。我们首先生成了一个2048位的RSA密钥对,并将公钥和私钥导出为字符串。然后定义了一个加密函数和一个解密函数,分别使用RSA加密算法和PKCS#1 OAEP填充方式进行加密和解密。最后我们测试了加解密的功能,并打印出了结果。
需要注意的是,这只是一个简单的PGP模拟公钥加解密示例,实际使用PGP时还需要考虑密钥管理、数字签名等问题。
阅读全文
相关推荐





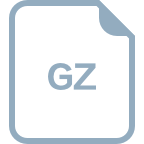







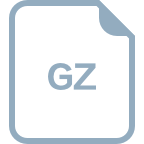

