把一本书的信息放在一个结构体变量中,然后输出这个书的信息。
时间: 2024-02-16 16:04:42 浏览: 17
好的,可以参考下面的代码实现:
```c
#include <stdio.h>
#include <string.h>
struct book {
char title[100];
char author[50];
char publisher[50];
int year;
};
int main() {
struct book mybook;
// 给结构体变量赋值
strcpy(mybook.title, "C语言程序设计");
strcpy(mybook.author, "谭浩强");
strcpy(mybook.publisher, "清华大学出版社");
mybook.year = 2019;
// 输出结构体变量中的信息
printf("书名:%s\n", mybook.title);
printf("作者:%s\n", mybook.author);
printf("出版社:%s\n", mybook.publisher);
printf("出版年份:%d\n", mybook.year);
return 0;
}
```
运行结果如下:
```
书名:C语言程序设计
作者:谭浩强
出版社:清华大学出版社
出版年份:2019
```
相关问题
把一个学生的信息(包括学号、姓名、性别、住址)放在一个结构体变量中,然后输出这个学生的信息。
以下是C语言的代码实现,将学生信息放在一个结构体变量中,然后输出这个学生的信息:
```c
#include <stdio.h>
int main() {
struct Student {
long int num;
char name[20];
char sex;
char addr[20];
} a = {10101, "Li Lin", 'M', "123 BeiJing Road"};
printf("NO.:%ld\nname:%s\nsex:%c\naddress:%s\n", a.num, a.name, a.sex, a.addr);
return 0;
}
```
输出结果为:
```
NO.:10101
name:Li Lin
sex:M
address:123 BeiJing Road
```
把一个学生的信息(包括学号、姓名、性别、住址)放在一个结构体变量中,然后输出这个学生的信息。
好的,下面是代码示例:
```c++
#include <iostream>
#include <string>
using namespace std;
// 定义学生结构体
struct Student {
int id; // 学号
string name; // 姓名
char gender; // 性别
string address; // 住址
};
int main() {
// 定义学生结构体变量并初始化
Student stu = { 20210001, "张三", 'M', "北京市海淀区" };
// 输出学生信息
cout << "学号:" << stu.id << endl;
cout << "姓名:" << stu.name << endl;
cout << "性别:" << stu.gender << endl;
cout << "住址:" << stu.address << endl;
return 0;
}
```
以上程序定义了一个名为 `Student` 的结构体,其中包含了学生的学号、姓名、性别和住址。在 `main` 函数中,定义了一个名为 `stu` 的结构体变量并初始化,然后输出了该学生的信息。
相关推荐
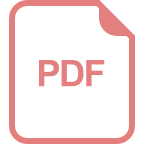
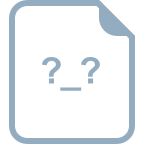
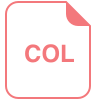











