用信号量解决经典同步问题和c语言代码示例
时间: 2024-02-21 07:00:55 浏览: 92
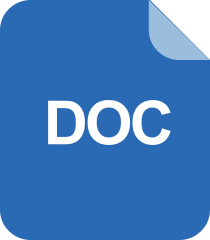
C语言信号量同步与互斥生产者消费者互斥锁读写者问题哲学家就餐问题课程设计

以下是使用信号量解决生产者-消费者问题的C语言代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <semaphore.h>
#define BUFFER_SIZE 10
int buffer[BUFFER_SIZE]; // 缓冲区
int in = 0; // 生产者放置数据的位置
int out = 0; // 消费者取走数据的位置
sem_t empty; // 空闲槽位数量
sem_t full; // 已经填充的槽位数量
pthread_mutex_t mutex; // 互斥访问缓冲区
void *producer(void *arg) {
int item;
while (1) {
item = rand() % 100; // 生产一个数据
sem_wait(&empty); // 等待空闲槽位
pthread_mutex_lock(&mutex); // 加锁
buffer[in] = item; // 放置数据
in = (in + 1) % BUFFER_SIZE; // 更新位置
printf("Producer produced item %d\n", item);
pthread_mutex_unlock(&mutex); // 解锁
sem_post(&full); // 发送已经填充的信号
sleep(rand() % 5); // 随机等待一段时间
}
}
void *consumer(void *arg) {
int item;
while (1) {
sem_wait(&full); // 等待已经填充的槽位
pthread_mutex_lock(&mutex); // 加锁
item = buffer[out]; // 取走数据
out = (out + 1) % BUFFER_SIZE; // 更新位置
printf("Consumer consumed item %d\n", item);
pthread_mutex_unlock(&mutex); // 解锁
sem_post(&empty); // 发送空闲槽位的信号
sleep(rand() % 5); // 随机等待一段时间
}
}
int main() {
pthread_t producer_thread, consumer_thread;
sem_init(&empty, 0, BUFFER_SIZE); // 初始化空闲槽位数量
sem_init(&full, 0, 0); // 初始化已经填充的槽位数量
pthread_mutex_init(&mutex, NULL); // 初始化互斥锁
pthread_create(&producer_thread, NULL, producer, NULL); // 创建生产者线程
pthread_create(&consumer_thread, NULL, consumer, NULL); // 创建消费者线程
pthread_join(producer_thread, NULL); // 等待生产者线程结束
pthread_join(consumer_thread, NULL); // 等待消费者线程结束
sem_destroy(&empty); // 销毁信号量
sem_destroy(&full);
pthread_mutex_destroy(&mutex); // 销毁互斥锁
return 0;
}
```
在代码中,生产者线程和消费者线程分别使用`sem_wait`和`sem_post`等函数等待和发送信号量,实现了对空闲槽位和已经填充的槽位数量的控制。同时,使用互斥锁保证了对缓冲区的互斥访问。
阅读全文
相关推荐
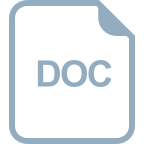
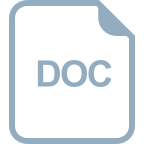






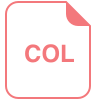








