编写程序删除单链表中所有关键字值为x的元素
时间: 2023-09-17 10:05:29 浏览: 173
### 回答1:
可以使用双指针法遍历单链表,找到关键字值为x的元素并删除。具体实现可以参考以下代码:
```python
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def removeElements(head: ListNode, val: int) -> ListNode:
dummy = ListNode(0)
dummy.next = head
prev, curr = dummy, head
while curr:
if curr.val == val:
prev.next = curr.next
else:
prev = curr
curr = curr.next
return dummy.next
```
其中,dummy节点用于处理头节点的删除情况,prev指针指向当前节点的前一个节点,curr指针指向当前节点。如果当前节点的值等于关键字值x,则将prev节点的next指针指向当前节点的下一个节点,否则将prev指针指向当前节点,curr指针指向下一个节点。最后返回dummy节点的next指针即可。
注意,这里使用了Python语言实现,如果使用其他语言需要进行相应的语法转换。
### 回答2:
编写程序删除单链表中所有关键字值为x的元素的思路如下:
1. 首先定义一个链表节点的结构体,包含一个整型的数据域和一个指向下一个节点的指针域。
2. 创建一个头节点(dummy node)作为链表的起始节点,方便处理删除时可能出现的头节点删除的情况。
3. 输入链表的长度n和关键字值x,并依次输入n个节点的数据值,构建单链表。
4. 遍历链表,找到第一个关键字值为x的节点,将上一个节点的指针域指向下一个节点,同时释放当前节点的内存空间。重复此步骤直到链表末尾。
5. 输出删除完成后的链表。
下面是一个用C语言编写的示例程序:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
Node* createLinkedList(int n) {
Node* head = (Node*)malloc(sizeof(Node));
head->next = NULL;
Node* current = head;
for (int i = 0; i < n; i++) {
int data;
scanf("%d", &data);
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
current->next = newNode;
current = newNode;
}
return head;
}
void deleteValue(Node* head, int x) {
Node* current = head;
while (current->next != NULL) {
if (current->next->data == x) {
Node* temp = current->next;
current->next = current->next->next;
free(temp);
} else {
current = current->next;
}
}
}
void printLinkedList(Node* head) {
Node* current = head->next;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
int main() {
int n, x;
printf("Input the length of the linked list: ");
scanf("%d", &n);
printf("Input the value to be deleted: ");
scanf("%d", &x);
printf("Input the elements of the linked list: ");
Node* head = createLinkedList(n);
deleteValue(head, x);
printf("Linked List after deletion: ");
printLinkedList(head);
return 0;
}
```
希望对您有帮助!
### 回答3:
为了编写程序删除单链表中所有关键字值为x的元素,首先需要定义一个单链表节点的结构体。
```C++
struct ListNode {
int val; // 存储节点的值
ListNode* next; // 指向下一个节点的指针
ListNode(int x) : val(x), next(nullptr) {}
};
```
接下来,可以使用迭代的方式遍历单链表,并删除关键字值为x的节点。具体的步骤如下:
1. 声明两个指针指向当前节点和前一个节点,初始时分别指向头节点和空指针。
2. 遍历单链表,直到当前节点为空。
3. 若当前节点的值为x,则删除当前节点。
- 将前一个节点的next指针指向当前节点的下一个节点。
- 释放当前节点的内存。
- 将当前节点指针指向下一个节点。
4. 若当前节点的值不为x,则将前一个节点指针指向当前节点,并将当前节点指针指向下一个节点。
5. 遍历结束后,返回头节点即可。
以下是具体的代码实现:
```C++
ListNode* removeElements(ListNode* head, int x) {
// 处理头节点为x的情况
while (head != nullptr && head->val == x) {
ListNode* temp = head;
head = head->next;
delete temp;
}
// 处理中间节点和尾节点为x的情况
ListNode* prev = nullptr; // 前一个节点
ListNode* curr = head; // 当前节点
while (curr != nullptr) {
if (curr->val == x) {
ListNode* temp = curr;
prev->next = curr->next;
curr = curr->next;
delete temp;
} else {
prev = curr;
curr = curr->next;
}
}
return head;
}
```
这样就完成了编写程序删除单链表中所有关键字值为x的元素的任务。
阅读全文
相关推荐
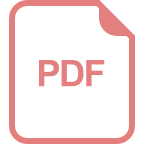

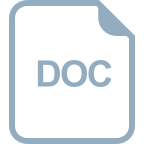
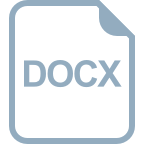
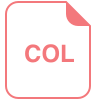
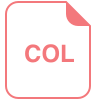
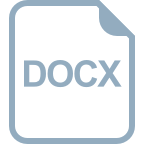
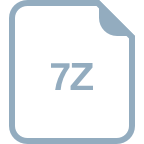
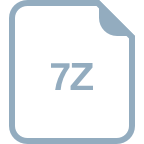
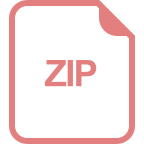