请编写springboot实现文件预览的代码
时间: 2023-04-04 20:01:34 浏览: 63
很高兴回答您的问题。以下是一个简单的 Spring Boot 实现文件预览的代码示例:
1. 首先,我们需要在 pom.xml 文件中添加以下依赖项:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.1.2</version>
</dependency>
```
2. 接下来,我们需要创建一个控制器类,用于处理文件预览请求:
```
@Controller
public class FilePreviewController {
@GetMapping("/preview")
public String previewFile(@RequestParam("file") MultipartFile file, Model model) throws IOException {
String fileName = file.getOriginalFilename();
String fileExtension = fileName.substring(fileName.lastIndexOf(".") + 1);
if (fileExtension.equalsIgnoreCase("docx")) {
XWPFDocument doc = new XWPFDocument(file.getInputStream());
XHTMLOptions options = XHTMLOptions.create();
ByteArrayOutputStream out = new ByteArrayOutputStream();
XHTMLConverter.getInstance().convert(doc, out, options);
model.addAttribute("content", new String(out.toByteArray(), "UTF-8"));
} else if (fileExtension.equalsIgnoreCase("xlsx")) {
XSSFWorkbook workbook = new XSSFWorkbook(file.getInputStream());
XSSFSheet sheet = workbook.getSheetAt();
DataFormatter dataFormatter = new DataFormatter();
StringBuilder sb = new StringBuilder();
for (Row row : sheet) {
for (Cell cell : row) {
String cellValue = dataFormatter.formatCellValue(cell);
sb.append(cellValue).append("\t");
}
sb.append("\n");
}
model.addAttribute("content", sb.toString());
} else {
model.addAttribute("content", "Unsupported file format");
}
return "preview";
}
}
```
3. 最后,我们需要创建一个 Thymeleaf 模板,用于显示预览内容:
```
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>File Preview</title>
</head>
<body>
<pre th:text="${content}"></pre>
</body>
</html>
```
以上就是一个简单的 Spring Boot 实现文件预览的代码示例。希望能对您有所帮助!
相关推荐
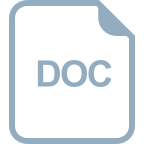
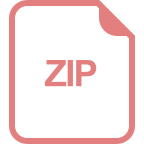
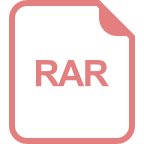
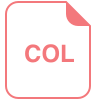
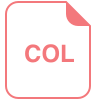
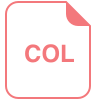
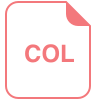
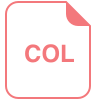









