用Python在EXCEL中实现先进先出成本核算代码
时间: 2024-05-03 16:18:47 浏览: 7
首先,我们需要使用Python的pandas库来读取和写入Excel文件。然后,我们可以使用pandas中的函数来进行先进先出成本核算。
以下是一个示例代码:
```python
import pandas as pd
# 读取Excel文件
df = pd.read_excel('inventory.xlsx')
# 计算每个产品的平均成本
df['average_cost'] = df['total_cost'] / df['quantity']
# 按照日期排序
df = df.sort_values(by=['date'])
# 初始化先进先出成本和销售额
fifo_cost = 0
sales_revenue = 0
# 遍历每个销售订单
for index, row in df.iterrows():
# 如果库存数量不足,则跳过该订单
if row['quantity'] > df['quantity'].sum():
continue
# 扣除库存数量
df.loc[index, 'quantity'] -= row['quantity']
# 计算销售额
sales_revenue += row['price'] * row['quantity']
# 计算先进先出成本
while row['quantity'] > 0:
# 获取最早进货的产品
earliest_row = df.iloc[0]
# 如果该产品库存数量不足,则删除该行
if earliest_row['quantity'] < row['quantity']:
fifo_cost += earliest_row['average_cost'] * earliest_row['quantity']
row['quantity'] -= earliest_row['quantity']
df = df.drop(earliest_row.name)
# 否则更新库存数量并计算成本
else:
fifo_cost += earliest_row['average_cost'] * row['quantity']
df.loc[earliest_row.name, 'quantity'] -= row['quantity']
row['quantity'] = 0
# 输出先进先出成本和销售额
print('FIFO cost:', fifo_cost)
print('Sales revenue:', sales_revenue)
```
此代码假设我们的Excel文件中有以下列:
- date:进货或销售日期
- product:产品名称
- quantity:进货或销售数量
- price:销售价格
- total_cost:进货总成本
输出将显示先进先出成本和销售额。请注意,此代码仅考虑一个产品的库存。如果需要进行多个产品的库存核算,请添加一个产品列,并在代码中相应地修改。
相关推荐
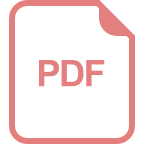
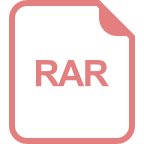
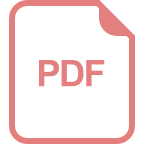














