pythonopencv视觉识别摆球角度
时间: 2023-10-11 08:11:20 浏览: 140
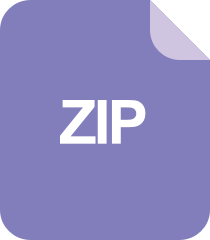
python+opencv运动目标检测

要实现摆球角度的视觉识别,可以使用OpenCV库。以下是一个简单的示例代码,该代码将摄像头捕获的图像进行处理,并计算出摆球的角度:
```python
import cv2
import numpy as np
# 捕获摄像头
cap = cv2.VideoCapture(0)
while True:
# 读取每一帧
ret, frame = cap.read()
# 转换为灰度图像
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 进行高斯滤波
blur = cv2.GaussianBlur(gray, (5, 5), 0)
# 进行边缘检测
edges = cv2.Canny(blur, 50, 150)
# 查找轮廓
contours, hierarchy = cv2.findContours(
edges, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 根据轮廓查找最小包围矩形
for contour in contours:
rect = cv2.minAreaRect(contour)
box = cv2.boxPoints(rect)
box = np.int0(box)
# 绘制矩形
cv2.drawContours(frame, [box], 0, (0, 0, 255), 2)
# 计算角度
angle = rect[2]
if angle < -45:
angle += 90
print("Angle:", angle)
# 显示图像
cv2.imshow("Frame", frame)
# 等待按键输入
key = cv2.waitKey(1) & 0xFF
# 按下 "q" 键退出循环
if key == ord("q"):
break
# 释放摄像头并关闭所有窗口
cap.release()
cv2.destroyAllWindows()
```
在上述代码中,首先使用 `cv2.VideoCapture()` 函数捕获摄像头。然后,将每一帧图像转换为灰度图像,并进行高斯滤波和边缘检测。接下来,使用 `cv2.findContours()` 函数查找轮廓,并使用 `cv2.minAreaRect()` 函数查找最小包围矩形。最后,计算出摆球的角度,并在图像上绘制矩形。
阅读全文
相关推荐

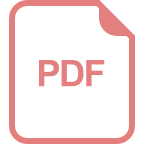
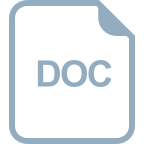
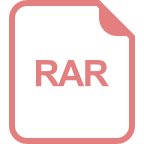
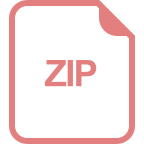
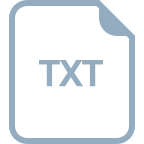
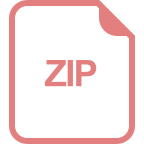
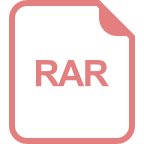
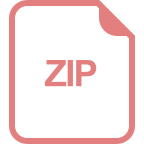
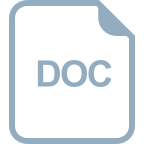
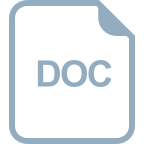
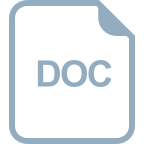
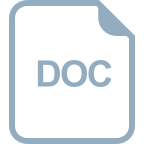
