白平衡,灰度世界算法(C# EmguCv)
时间: 2023-06-30 11:06:33 浏览: 192
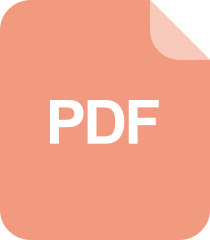
C# EmguCV应用

白平衡是一种用于调整图像颜色的方法,它可以使图像中的白色看起来更加真实和自然。在数字图像处理中,常用的白平衡算法有基于灰度世界假设的算法。
灰度世界算法假设整个图像中的颜色分布是均匀的,因此平均颜色应该是灰色。该算法的步骤如下:
1. 计算整个图像的 RGB 三个通道的平均值。
2. 计算每个通道的增益因子,使得每个通道的平均值等于整个图像的平均值。
3. 将每个像素的 RGB 值乘以对应通道的增益因子,以实现白平衡。
以下是使用 EmguCv 库实现灰度世界算法的 C# 代码示例:
```csharp
using Emgu.CV;
using Emgu.CV.Structure;
public static Image<Bgr, byte> GrayWorld(Image<Bgr, byte> img)
{
// 计算图像的平均 B、G、R 值
double avgB = 0, avgG = 0, avgR = 0;
int pixelCount = img.Width * img.Height;
for (int y = 0; y < img.Height; y++)
{
for (int x = 0; x < img.Width; x++)
{
Bgr color = img[y, x];
avgB += color.Blue;
avgG += color.Green;
avgR += color.Red;
}
}
avgB /= pixelCount;
avgG /= pixelCount;
avgR /= pixelCount;
// 计算每个通道的增益因子
double[] gain = new double[3];
gain[0] = avgG / avgB; // 蓝色通道的增益因子
gain[1] = 1.0; // 绿色通道的增益因子
gain[2] = avgG / avgR; // 红色通道的增益因子
// 对每个像素应用增益因子
for (int y = 0; y < img.Height; y++)
{
for (int x = 0; x < img.Width; x++)
{
Bgr color = img[y, x];
byte newB = (byte)(color.Blue * gain[0]);
byte newG = (byte)(color.Green * gain[1]);
byte newR = (byte)(color.Red * gain[2]);
img[y, x] = new Bgr(newB, newG, newR);
}
}
return img;
}
```
使用方法:
```csharp
Image<Bgr, byte> img = new Image<Bgr, byte>("image.jpg");
img = GrayWorld(img);
img.Save("result.jpg");
```
阅读全文
相关推荐
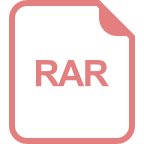
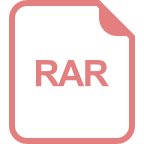















