设计一个形状类 Shape,包含一个 getArea()方法,该方法不包含实际语句。在 Shape 类基础上设计圆形、矩形、三角形和梯形四个子类,要求根据实际形状重写 getArea()方法。设计一个 TestShape 类,包含变量 area(存储总面积)、静态方法 countArea(Shape s),该方法负责把参数中的形状面积加入到 area 中。在 main 函数中新建(2)中四种类型的对象s1、s2、s3、s4,通过调用 countArea 方法把四个对象面积累加到 area 中,最后输出 area。
时间: 2024-03-20 14:43:13 浏览: 73
好的,这是一个关于面向对象的问题,我会为您解答。
首先,我们先定义 Shape 类,代码如下:
```java
public class Shape {
public double getArea() {
return 0;
}
}
```
Shape 类中只有一个 getArea() 方法,该方法没有实际语句,因为我们在子类中会重写该方法,实现不同形状的面积计算。
接下来,我们分别定义圆形、矩形、三角形和梯形四个子类,代码如下:
```java
public class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double getArea() {
return Math.PI * radius * radius;
}
}
public class Rectangle extends Shape {
private double length;
private double width;
public Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
@Override
public double getArea() {
return length * width;
}
}
public class Triangle extends Shape {
private double base;
private double height;
public Triangle(double base, double height) {
this.base = base;
this.height = height;
}
@Override
public double getArea() {
return 0.5 * base * height;
}
}
public class Trapezoid extends Shape {
private double top;
private double bottom;
private double height;
public Trapezoid(double top, double bottom, double height) {
this.top = top;
this.bottom = bottom;
this.height = height;
}
@Override
public double getArea() {
return 0.5 * (top + bottom) * height;
}
}
```
可以看出,每个子类都继承了 Shape 类,并重写了其中的 getArea() 方法,根据实际形状计算出面积。
最后,我们定义 TestShape 类,实现面积计算和输出,代码如下:
```java
public class TestShape {
private static double area = 0;
public static void countArea(Shape s) {
area += s.getArea();
}
public static void main(String[] args) {
Shape s1 = new Circle(5);
Shape s2 = new Rectangle(3, 4);
Shape s3 = new Triangle(2, 6);
Shape s4 = new Trapezoid(3, 5, 4);
countArea(s1);
countArea(s2);
countArea(s3);
countArea(s4);
System.out.println("Total area: " + area);
}
}
```
在 main 函数中,我们新建了四个不同形状的对象 s1、s2、s3、s4,然后通过 countArea 方法,将每个对象的面积累加到静态变量 area 中,最后输出总面积。
希望能够对您有所帮助!
阅读全文
相关推荐
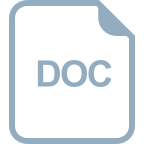
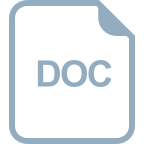
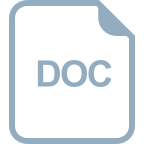









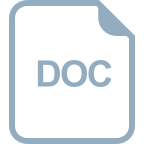





