请用C++语言给出一个程序设计模式中的简单工厂模式
时间: 2024-05-03 18:22:46 浏览: 8
简单工厂模式是一种创建型设计模式,它提供了一种创建对象的最佳实践。在简单工厂模式中,我们使用一个工厂类根据传递给它的参数来创建不同的对象。
以下是一个使用简单工厂模式创建不同形状的程序:
```c
#include <stdio.h>
/* 形状的基类 */
typedef struct {
void (*draw)(void); // 绘制形状的函数指针
} Shape;
/* 圆形 */
typedef struct {
Shape shape; // 继承 Shape 基类
} Circle;
void drawCircle(void) {
printf("Drawing a circle.\n");
}
/* 矩形 */
typedef struct {
Shape shape; // 继承 Shape 基类
} Rectangle;
void drawRectangle(void) {
printf("Drawing a rectangle.\n");
}
/* 工厂类 */
Shape* createShape(char type) {
switch (type) {
case 'C':
Circle* circle = (Circle*)malloc(sizeof(Circle));
circle->shape.draw = drawCircle;
return (Shape*)circle;
case 'R':
Rectangle* rect = (Rectangle*)malloc(sizeof(Rectangle));
rect->shape.draw = drawRectangle;
return (Shape*)rect;
default:
return NULL;
}
}
int main() {
Shape* shape1 = createShape('C');
shape1->draw(); // Drawing a circle.
Shape* shape2 = createShape('R');
shape2->draw(); // Drawing a rectangle.
return 0;
}
```
在上面的程序中,我们定义了一个基类 `Shape`,和两个派生类 `Circle` 和 `Rectangle`,它们都继承自 `Shape` 基类。每个形状类都有一个 `draw` 函数指针,用于绘制该形状。
我们还定义了一个工厂类 `createShape`,它根据传递给它的参数来创建不同的形状对象。在 `main` 函数中,我们使用工厂类创建了一个圆形和一个矩形,然后分别调用它们的 `draw` 函数来绘制它们。
这就是简单工厂模式的基本实现。通过使用此模式,我们可以将对象的创建与客户端代码分离开来,从而使代码更具可维护性和可扩展性。
相关推荐
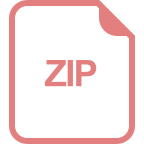














