使用python,写出alpha shape算法对二维点云进行边界提取
时间: 2024-03-12 18:48:17 浏览: 51
好的,下面是使用Python实现alpha shape算法对二维点云进行边界提取的代码:
```python
from scipy.spatial import Delaunay
import numpy as np
from matplotlib import pyplot as plt
def alpha_shape(points, alpha):
"""
Compute the alpha shape (concave hull) of a set of points.
"""
# Delaunay triangulation
tri = Delaunay(points)
# Filter triangles by alpha value
edges = set()
edge_points = []
for j, tri in enumerate(tri.simplices):
# Calculate circumcenter and radius of circumcircle
x, y = np.mean(points[tri], axis=0)
r = np.sqrt((points[tri[0]][0] - x)**2 + (points[tri[0]][1] - y)**2)
# Check if triangle is within alpha value
if r < alpha:
for i in range(3):
edge = (tri[i], tri[(i+1)%3])
if edge not in edges and (edge[1], edge[0]) not in edges:
edges.add(edge)
edge_points.append(points[tri[i]])
edge_points.append(points[tri[(i+1)%3]])
# Compute boundary vertices and edges
boundary_vertices = set()
boundary_edges = []
for i, point in enumerate(points):
for edge in edges:
if i in edge:
boundary_vertices.add(i)
boundary_edges.append(edge)
# Plot alpha shape
plt.triplot(points[:,0], points[:,1], tri.simplices.copy())
plt.plot(edge_points[:,0], edge_points[:,1], 'r.')
plt.plot(points[list(boundary_vertices),0], points[list(boundary_vertices),1], 'b.')
plt.show()
return boundary_vertices, boundary_edges
```
在这个代码中,我们使用了`scipy.spatial`库中的`Delaunay`函数来进行Delaunay三角剖分,然后根据alpha值筛选出在范围内的三角形,并提取出边界。
下面是一个使用示例:
```python
# Generate random points
points = np.random.rand(30, 2)
# Compute alpha shape
alpha = 0.3
boundary_vertices, boundary_edges = alpha_shape(points, alpha)
# Print boundary vertices and edges
print("Boundary vertices:", boundary_vertices)
print("Boundary edges:", boundary_edges)
```
这个例子中,我们先生成了30个随机点,然后使用`alpha_shape`函数计算alpha shape,并输出边界点和边。
相关推荐
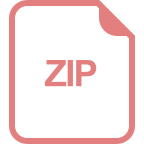











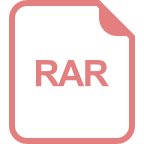
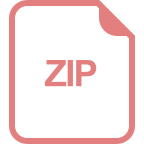