用C++语言编写程序,基于字符串实现对超长整数的加法运算和减法运算,输入两个超长整数和运算符(+或-),输出运算结果。
时间: 2024-03-17 22:46:14 浏览: 114
好的,以下是基于 C++ 语言实现的代码,可以实现超长整数的加法和减法运算:
```cpp
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;
// 去除字符串前导0
string removeLeadingZeros(string num_str) {
num_str.erase(0, num_str.find_first_not_of('0'));
if (num_str.empty()) {
return "0";
}
else {
return num_str;
}
}
// 超长整数加法
string add(string num1, string num2) {
// 先把两个数对齐
int len1 = num1.length(), len2 = num2.length();
if (len1 > len2) {
num2 = string(len1 - len2, '0') + num2;
}
else if (len1 < len2) {
num1 = string(len2 - len1, '0') + num1;
}
// 从低位向高位按位相加
int carry = 0; // 进位
string res;
for (int i = len1 - 1; i >= 0; i--) {
int n1 = num1[i] - '0', n2 = num2[i] - '0';
int s = n1 + n2 + carry;
carry = s / 10;
res += to_string(s % 10);
}
if (carry) {
res += to_string(carry);
}
// 把结果反转并转成字符串
reverse(res.begin(), res.end());
return res;
}
// 超长整数减法
string sub(string num1, string num2) {
// 先比较被减数和减数的大小,如果被减数小于减数,则交换被减数和减数,并加上负号
bool negative = false;
if (num1.length() < num2.length() || (num1.length() == num2.length() && num1 < num2)) {
swap(num1, num2);
negative = true;
}
// 先把两个数对齐
int len1 = num1.length(), len2 = num2.length();
if (len1 > len2) {
num2 = string(len1 - len2, '0') + num2;
}
else if (len1 < len2) {
num1 = string(len2 - len1, '0') + num1;
}
// 从低位向高位按位相减
int borrow = 0; // 借位
string res;
for (int i = len1 - 1; i >= 0; i--) {
int n1 = num1[i] - '0', n2 = num2[i] - '0';
int s = n1 - n2 - borrow;
if (s < 0) {
s += 10;
borrow = 1;
}
else {
borrow = 0;
}
res += to_string(s);
}
// 去除结果前导0并加上负号(如果有的话)
res = removeLeadingZeros(res);
if (negative) {
res = '-' + res;
}
return res;
}
int main() {
string num1, num2, op;
cout << "请输入第一个超长整数:";
cin >> num1;
cout << "请输入第二个超长整数:";
cin >> num2;
cout << "请输入运算符(+或-):";
cin >> op;
string res;
if (op == "+") {
res = add(num1, num2);
}
else if (op == "-") {
res = sub(num1, num2);
}
else {
cout << "不支持的运算符" << endl;
return 0;
}
cout << "运算结果:" << res << endl;
return 0;
}
```
该程序首先定义了两个函数:`removeLeadingZeros`、`add`、`sub`。其中 `removeLeadingZeros` 函数用于去除字符串的前导0,`add` 函数实现超长整数的加法运算,`sub` 函数实现超长整数的减法运算。
最后在主函数中,程序会获取用户输入的超长整数表达式,并根据运算符调用 `add` 或 `sub` 函数进行计算,并输出运算结果。
阅读全文
相关推荐
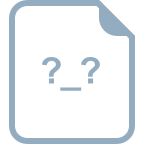
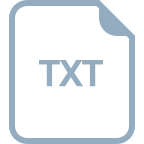
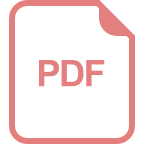









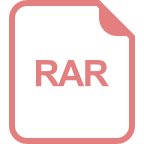
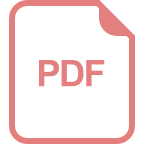
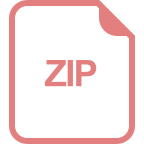
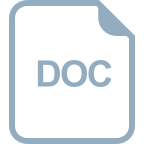
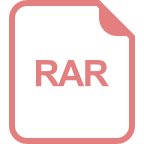
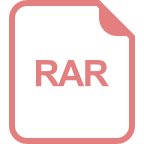
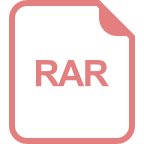