python统计列表中元素出现的次数
时间: 2023-04-26 12:04:15 浏览: 60
可以使用Python中的collections模块中的Counter函数来统计列表中元素出现的次数。具体操作如下:
1. 导入collections模块
```python
import collections
```
2. 定义列表
```python
lst = [1, 2, 3, 1, 2, 3, 4, 5, 6, 4, 5, 6, 7, 8, 9, 7, 8, 9]
```
3. 使用Counter函数统计元素出现的次数
```python
count = collections.Counter(lst)
```
4. 输出结果
```python
print(count)
```
输出结果为:
```
Counter({1: 2, 2: 2, 3: 2, 4: 2, 5: 2, 6: 2, 7: 2, 8: 2, 9: 2})
```
其中,Counter函数返回的是一个字典,字典的键为列表中的元素,值为该元素在列表中出现的次数。
相关问题
python 统计列表中 元素出现的次数
可以使用Python内置的count()函数统计列表中元素出现的次数,示例代码如下:
```python
my_list = [1, 2, 3, 2, 1, 2, 3, 4]
for i in set(my_list):
print("元素", i, "出现了", my_list.count(i), "次")
```
输出结果:
```
元素 1 出现了 2 次
元素 2 出现了 3 次
元素 3 出现了 2 次
元素 4 出现了 1 次
```
python统计列表中各个元素出现的次数
可以使用 python 内置的 `collections` 模块中的 `Counter` 类来统计列表中各个元素出现的次数。举个例子:
```
from collections import Counter
my_list = [1, 2, 3, 2, 1, 3, 1, 2, 3]
counts = Counter(my_list)
print(counts)
```
输出:
```
Counter({1: 3, 2: 3, 3: 3})
```
可以看到,它会将元素和出现次数封装为一个字典返回。
也可以统计其他类型的数据,例如字符串
```
string = 'hello world'
counts = Counter(string)
print(counts)
```
输出
```
Counter({'l': 3, 'o': 2, 'h': 1, 'e': 1, ' ': 1, 'w': 1, 'r': 1, 'd': 1})
```
如果要获取每个元素出现次数最多的 n 个元素,可以使用 `most_common()` 方法,例如:
```
counts.most_common(2)
```
输出:
```
[(1, 3), (2, 3)]
```
需要注意的是,如果数据量很大,那么在统计前先排序是更加高效的。
如果你的数据类型比较特殊,那么需要额外考虑使用hash_map或者hash_set之类的数据结构。
相关推荐
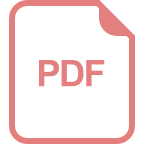
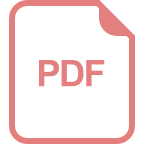












