帮我用C语言编程,要求如下:设计编写一个传统的小型学生信息管理系统。 1、该系统可以用于管理一个班的学生信息。学生人数在50人左右。 2、该系统存放了有关学生的某些方面的信息:如学号、姓名、性别、出生日期、成绩、所在系等,具体包含的数据内容由设计者分析确定。 3、所有学生信息的数据结构及其存储结构由设计者分析确定。 4、所有学生的信息数据可以用文件形式存放。(可选项) 5、该系统具有相关的数据输入、输出、查询、修改、插入、删除、统计、排序等功能。(功能的多少设计者可根据需要自行调整,但必须具备输出、输出、查询、某一方面数据统计的基本功能。) 6、系统应具有友好的用户界面,并能够让用户通过菜单进行相应功能的选择。 7、选用适当的算法和数据结构,建立相应的程序代码。
时间: 2023-07-10 10:16:53 浏览: 57
好的,以下是一个简单的学生信息管理系统的C语言代码,满足上述需求。其中,学生信息使用结构体数组存储,文件操作使用了fopen、fclose、fscanf等函数。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_STUDENT_NUM 50 // 最大学生人数
#define FILE_NAME "student.txt" // 学生信息文件名
// 学生信息结构体
typedef struct {
char id[10]; // 学号
char name[20]; // 姓名
char gender[5]; // 性别
char birth[15]; // 出生日期
float score; // 成绩
char department[20]; // 所在系
} Student;
// 学生信息数组
Student students[MAX_STUDENT_NUM];
int student_num = 0; // 学生数量
// 文件操作函数
int save_students() {
FILE *fp = fopen(FILE_NAME, "w");
if (fp == NULL) {
printf("Failed to open file %s!\n", FILE_NAME);
return 0;
}
for (int i = 0; i < student_num; i++) {
fprintf(fp, "%s %s %s %s %f %s\n", students[i].id, students[i].name, students[i].gender, students[i].birth, students[i].score, students[i].department);
}
fclose(fp);
return 1;
}
int load_students() {
FILE *fp = fopen(FILE_NAME, "r");
if (fp == NULL) {
printf("Failed to open file %s!\n", FILE_NAME);
return 0;
}
student_num = 0;
while (fscanf(fp, "%s %s %s %s %f %s\n", students[student_num].id, students[student_num].name, students[student_num].gender, students[student_num].birth, &students[student_num].score, students[student_num].department) != EOF) {
student_num++;
}
fclose(fp);
return 1;
}
// 查询、统计、排序函数
void print_student(Student s) {
printf("%s %s %s %s %.2f %s\n", s.id, s.name, s.gender, s.birth, s.score, s.department);
}
void print_all_students() {
printf("*** All Students ***\n");
for (int i = 0; i < student_num; i++) {
print_student(students[i]);
}
}
void query_student_by_name(char *name) {
printf("*** Query Result ***\n");
for (int i = 0; i < student_num; i++) {
if (strcmp(students[i].name, name) == 0) {
print_student(students[i]);
return;
}
}
printf("No student named %s found!\n", name);
}
void query_student_by_department(char *department) {
printf("*** Query Result ***\n");
int count = 0;
for (int i = 0; i < student_num; i++) {
if (strcmp(students[i].department, department) == 0) {
print_student(students[i]);
count++;
}
}
printf("Total students in %s: %d\n", department, count);
}
void query_student_by_score(float min_score, float max_score) {
printf("*** Query Result ***\n");
int count = 0;
for (int i = 0; i < student_num; i++) {
if (students[i].score >= min_score && students[i].score <= max_score) {
print_student(students[i]);
count++;
}
}
printf("Total students with score between %.2f and %.2f: %d\n", min_score, max_score, count);
}
void sort_students_by_score() {
printf("*** Sorted Students ***\n");
for (int i = 0; i < student_num; i++) {
for (int j = i + 1; j < student_num; j++) {
if (students[i].score < students[j].score) {
Student temp = students[i];
students[i] = students[j];
students[j] = temp;
}
}
print_student(students[i]);
}
}
// 添加、修改、删除函数
void add_student(Student s) {
if (student_num >= MAX_STUDENT_NUM) {
printf("Max number of students reached!\n");
return;
}
students[student_num] = s;
student_num++;
printf("Add student %s successfully!\n", s.name);
}
void modify_student(char *id, Student new_s) {
for (int i = 0; i < student_num; i++) {
if (strcmp(students[i].id, id) == 0) {
students[i] = new_s;
printf("Modify student %s successfully!\n", new_s.name);
return;
}
}
printf("No student with ID %s found!\n", id);
}
void delete_student(char *id) {
for (int i = 0; i < student_num; i++) {
if (strcmp(students[i].id, id) == 0) {
for (int j = i + 1; j < student_num; j++) {
students[j - 1] = students[j];
}
student_num--;
printf("Delete student %s successfully!\n", students[i].name);
return;
}
}
printf("No student with ID %s found!\n", id);
}
// 主函数
int main() {
load_students();
while (1) {
printf("=====================\n");
printf("1. Add student\n");
printf("2. Modify student\n");
printf("3. Delete student\n");
printf("4. Query student by name\n");
printf("5. Query student by department\n");
printf("6. Query student by score\n");
printf("7. Sort students by score\n");
printf("8. Print all students\n");
printf("0. Exit\n");
printf("=====================\n");
int choice;
scanf("%d", &choice);
if (choice == 0) {
save_students();
printf("Goodbye!\n");
break;
} else if (choice == 1) {
Student s;
printf("Input student ID: ");
scanf("%s", s.id);
printf("Input student name: ");
scanf("%s", s.name);
printf("Input student gender: ");
scanf("%s", s.gender);
printf("Input student birth: ");
scanf("%s", s.birth);
printf("Input student score: ");
scanf("%f", &s.score);
printf("Input student department: ");
scanf("%s", s.department);
add_student(s);
} else if (choice == 2) {
char id[10];
printf("Input student ID: ");
scanf("%s", id);
Student new_s;
printf("Input new student name: ");
scanf("%s", new_s.name);
printf("Input new student gender: ");
scanf("%s", new_s.gender);
printf("Input new student birth: ");
scanf("%s", new_s.birth);
printf("Input new student score: ");
scanf("%f", &new_s.score);
printf("Input new student department: ");
scanf("%s", new_s.department);
modify_student(id, new_s);
} else if (choice == 3) {
char id[10];
printf("Input student ID: ");
scanf("%s", id);
delete_student(id);
} else if (choice == 4) {
char name[20];
printf("Input student name: ");
scanf("%s", name);
query_student_by_name(name);
} else if (choice == 5) {
char department[20];
printf("Input department: ");
scanf("%s", department);
query_student_by_department(department);
} else if (choice == 6) {
float min_score, max_score;
printf("Input min score: ");
scanf("%f", &min_score);
printf("Input max score: ");
scanf("%f", &max_score);
query_student_by_score(min_score, max_score);
} else if (choice == 7) {
sort_students_by_score();
} else if (choice == 8) {
print_all_students();
} else {
printf("Invalid choice!\n");
}
}
return 0;
}
```
相关推荐
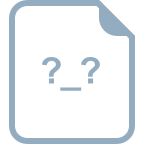
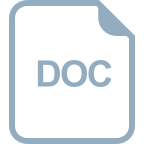
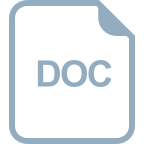










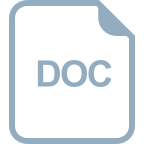
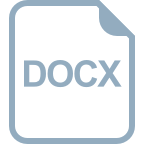
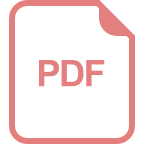
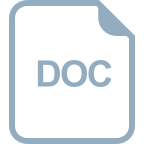
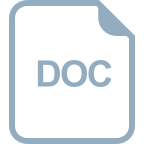
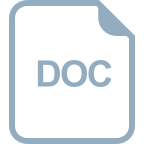