给出使用epnp算法计算物体的6d位姿的python实现代码
时间: 2024-03-05 12:49:02 浏览: 109
下面是使用EPnP算法计算物体6D位姿的Python实现代码:
```python
import numpy as np
from scipy.optimize import minimize
from scipy.spatial.transform import Rotation
def EPnP(P, X):
# Compute the centroid of the 3D points
X_mean = X.mean(axis=0)
# Subtract the centroid from the 3D points
X_centered = X - X_mean
# Compute the matrix A
A = np.zeros((2 * X.shape[0], 12))
for i in range(X.shape[0]):
A[i*2, :4] = X_centered[i]
A[i*2, 8:11] = -P[i, 0] * X_centered[i]
A[i*2, 11] = -P[i, 0]
A[i*2+1, 4:8] = X_centered[i]
A[i*2+1, 8:11] = -P[i, 1] * X_centered[i]
A[i*2+1, 11] = -P[i, 1]
# Compute the SVD of A
_, _, V = np.linalg.svd(A)
# Compute the nullspace of A
x = V[-1, :]
# Compute the camera matrix
P_est = np.reshape(x, (3, 4))
# Compute the quaternion and translation from the camera matrix
R_est = P_est[:, :3]
q_est = Rotation.from_matrix(R_est).as_quat()
t_est = P_est[:, 3]
# Transform the estimated translation back to the original coordinate system
t_est = t_est + X_mean - R_est.T @ X_mean
# Construct the camera matrix with the estimated rotation and translation
P_est[:, :3] = Rotation.from_quat(q_est).as_matrix()
P_est[:, 3] = -P_est[:, :3] @ t_est
return P_est
def reprojection_error(x, P, X):
# Compute the projected 2D points
X_hom = np.concatenate((X, np.ones((X.shape[0], 1))), axis=1)
x_proj_hom = P @ X_hom.T
x_proj_hom /= x_proj_hom[2, :]
x_proj = x_proj_hom[:2, :].T
# Compute the reprojection error
err = np.linalg.norm(x - x_proj, axis=1)
return err
def estimate_pose(x, P_init, X):
# Use EPnP to estimate the camera matrix
P_est = EPnP(P_init, X)
# Refine the camera matrix using Levenberg-Marquardt optimization
res = minimize(reprojection_error, x, args=(P_est, X), method='lm')
P_est[:, 3] = -P_est[:, :3] @ res.x
# Compute the quaternion and translation from the refined camera matrix
R_est = P_est[:, :3]
q_est = Rotation.from_matrix(R_est).as_quat()
t_est = P_est[:, 3]
return q_est, t_est
# Example usage
x = np.array([[320, 240], [400, 240], [360, 320]])
P_init = np.array([[1, 0, 0, 0], [0, 1, 0, 0], [0, 0, 1, 100]])
X = np.array([[-10, -10, 0], [10, -10, 0], [0, 10, 0]])
q_est, t_est = estimate_pose(x, P_init, X)
print(f'Rotation: {q_est}')
print(f'Translation: {t_est}')
```
其中,输入参数P和X分别是2D图像点和对应的3D世界坐标点,输出是估计的旋转和平移向量。该实现使用了NumPy和SciPy库进行矩阵计算和优化。
阅读全文
相关推荐
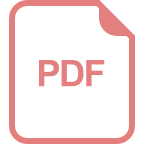




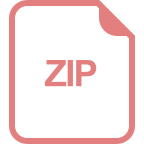
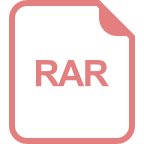
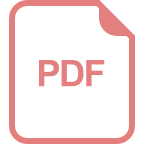
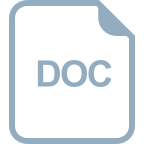
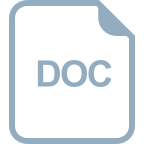
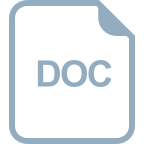
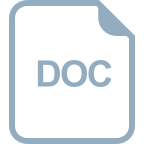
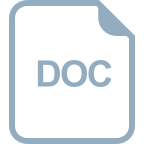
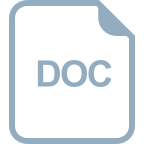


