idea中配置socket项目环境
时间: 2023-04-07 18:00:38 浏览: 111
可以回答这个问题。在IDEA中配置socket项目环境,需要先创建一个Java项目,然后在项目中添加socket相关的依赖库,例如Java的Socket类库和Netty框架等。接着,在代码中编写socket相关的逻辑,例如创建socket连接、发送和接收数据等。最后,可以通过IDEA的调试功能来测试和调试socket项目。
相关问题
idea配置socket项目环境
我可以回答这个问题。首先,你需要在你的IDEA中创建一个新的Java项目。然后,你需要添加Java Socket库到你的项目中。接下来,你需要编写你的Socket代码,并将其与你的项目一起编译。最后,你可以运行你的Socket程序并测试它是否正常工作。希望这可以帮助你配置你的Socket项目环境。
用idea使用windows socket 编写C/S程序,具有简单的远程控制功能,给出完整详细可运行代码
在 IntelliJ IDEA 中编写一个简单的 Windows Socket C/S(客户端/服务器)程序用于远程控制,我们首先需要设置环境并理解基本的概念。Windows Socket 提供了 TCP/IP 协议族的通信支持。以下是一个基础的示例:
**服务器端 (Server.java)**
```java
import java.io.*;
import java.net.*;
public class Server {
private static final int PORT = 8000;
public static void main(String[] args) throws IOException {
ServerSocket serverSocket = new ServerSocket(PORT);
System.out.println("Server started on port " + PORT);
while (true) {
Socket clientSocket = serverSocket.accept();
new Thread(new ClientHandler(clientSocket)).start();
}
}
private static class ClientHandler implements Runnable {
private Socket clientSocket;
public ClientHandler(Socket clientSocket) {
this.clientSocket = clientSocket;
}
@Override
public void run() {
try (
BufferedReader in = new BufferedReader(
new InputStreamReader(clientSocket.getInputStream())
);
PrintWriter out = new PrintWriter(clientSocket.getOutputStream(), true)
) {
String inputLine;
while ((inputLine = in.readLine()) != null) {
System.out.println("Received command: " + inputLine);
// 这里可以根据输入执行相应的操作,比如修改文件、关闭进程等
if ("exit".equals(inputLine)) {
break;
}
out.println("Command executed");
}
clientSocket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
**客户端 (Client.java)**
```java
import java.io.*;
import java.net.*;
public class Client {
public static void main(String[] args) throws IOException {
String host = "localhost";
int port = 8000;
Socket clientSocket = new Socket(host, port);
BufferedReader in = new BufferedReader(new InputStreamReader(clientSocket.getInputStream()));
PrintWriter out = new PrintWriter(clientSocket.getOutputStream(), true);
BufferedReader stdIn = new BufferedReader(new InputStreamReader(System.in));
String userInput;
System.out.println("Connected to the server");
while ((userInput = stdIn.readLine()) != null) {
out.println(userInput); // 发送命令到服务器
System.out.println("Sent: " + userInput);
if ("exit".equals(userInput)) {
break;
}
String response = in.readLine(); // 接收服务器响应
System.out.println("Response from server: " + response);
}
clientSocket.close();
}
}
```
在这个例子中,客户端通过 `Socket` 连接到服务器,并发送字符串指令。服务器端监听指定端口,接受客户端连接并处理请求。
**注意事项**:
1. 由于安全性考虑,实际项目中应使用 SSL/TLS 加密连接。
2. 远程控制功能通常涉及权限管理和安全措施,防止恶意攻击。
**相关问题--:**
1. 在实际应用中如何处理错误和异常?
2. 如何在客户端添加身份验证机制?
3. 如何在服务器端实现更复杂的命令解析和执行逻辑?
阅读全文
相关推荐
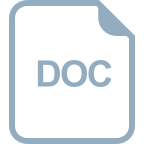
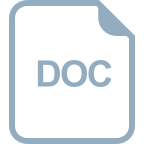
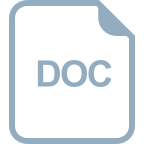
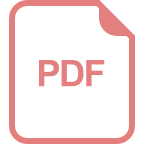
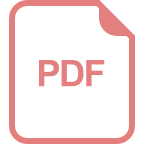
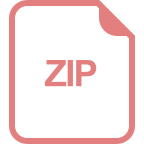
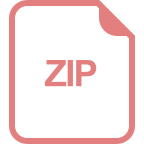
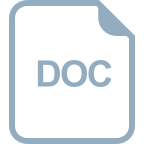
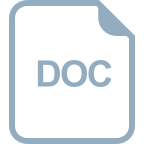
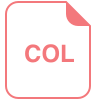
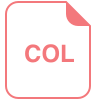
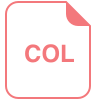
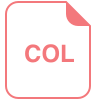



