用PYTHON写一个基于tushare数据的中证1000指数的VAR和CVAR的风险预警模型
时间: 2024-05-06 20:20:04 浏览: 12
首先,需要安装tushare库,并导入所需的库和模块:
```python
import tushare as ts
import numpy as np
import pandas as pd
from scipy.stats import norm
import matplotlib.pyplot as plt
```
然后,获取中证1000指数的历史数据:
```python
index_data = ts.get_k_data('000852', start='2010-01-01', end='2021-12-31')
index_data.set_index('date', inplace=True)
index_data.sort_index(inplace=True)
index_data.head()
```
接下来,计算日收益率:
```python
returns = np.log(index_data['close'] / index_data['close'].shift(1))
returns.dropna(inplace=True)
```
计算历史VAR和CVAR:
```python
alpha = 0.05 # 设置置信水平为0.05
var = norm.ppf(alpha) * returns.std()
cvar = returns[returns <= -var].mean()
```
接下来,定义风险预警函数:
```python
def risk_warning():
last_price = ts.get_realtime_quotes('000852')['price'].iloc[0] # 获取最新价格
last_return = np.log(float(last_price) / index_data['close'].iloc[-1])
var_warning = index_data['close'].iloc[-1] * (1 - np.exp(-var * np.sqrt(1))) # VAR风险预警价位
cvar_warning = index_data['close'].iloc[-1] * (1 - np.exp(-cvar * np.sqrt(1))) # CVAR风险预警价位
if float(last_price) <= var_warning:
print('VAR风险预警!最新价格为%s,VAR风险预警价位为%s。' % (last_price, round(var_warning, 2)))
elif float(last_price) <= cvar_warning:
print('CVAR风险预警!最新价格为%s,CVAR风险预警价位为%s。' % (last_price, round(cvar_warning, 2)))
else:
print('风险正常。最新价格为%s,VAR风险预警价位为%s,CVAR风险预警价位为%s。' % (last_price, round(var_warning, 2), round(cvar_warning, 2)))
```
最后,调用函数进行风险预警:
```python
risk_warning()
```
完整代码如下:
```python
import tushare as ts
import numpy as np
import pandas as pd
from scipy.stats import norm
import matplotlib.pyplot as plt
index_data = ts.get_k_data('000852', start='2010-01-01', end='2021-12-31')
index_data.set_index('date', inplace=True)
index_data.sort_index(inplace=True)
returns = np.log(index_data['close'] / index_data['close'].shift(1))
returns.dropna(inplace=True)
alpha = 0.05 # 设置置信水平为0.05
var = norm.ppf(alpha) * returns.std()
cvar = returns[returns <= -var].mean()
def risk_warning():
last_price = ts.get_realtime_quotes('000852')['price'].iloc[0] # 获取最新价格
last_return = np.log(float(last_price) / index_data['close'].iloc[-1])
var_warning = index_data['close'].iloc[-1] * (1 - np.exp(-var * np.sqrt(1))) # VAR风险预警价位
cvar_warning = index_data['close'].iloc[-1] * (1 - np.exp(-cvar * np.sqrt(1))) # CVAR风险预警价位
if float(last_price) <= var_warning:
print('VAR风险预警!最新价格为%s,VAR风险预警价位为%s。' % (last_price, round(var_warning, 2)))
elif float(last_price) <= cvar_warning:
print('CVAR风险预警!最新价格为%s,CVAR风险预警价位为%s。' % (last_price, round(cvar_warning, 2)))
else:
print('风险正常。最新价格为%s,VAR风险预警价位为%s,CVAR风险预警价位为%s。' % (last_price, round(var_warning, 2), round(cvar_warning, 2)))
risk_warning()
```
相关推荐
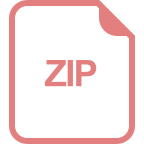
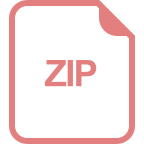
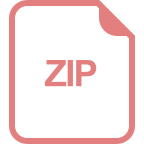














