for item in set_Global_Time: id = [i for i, x in enumerate(Global_Time) if x == item]
时间: 2024-05-23 19:11:14 浏览: 14
这段代码使用了列表推导式和enumerate函数。它的作用是根据一个给定的列表Global_Time,找出其中所有等于set_Global_Time中的元素的下标,并将这些下标存储在列表id中。
具体的解释如下:
- set_Global_Time是一个集合,包含了一些时间值。
- 在循环中,item代表set_Global_Time中的每一个元素。
- 列表推导式[i for i, x in enumerate(Global_Time) if x == item],意味着对于Global_Time列表中的每一个元素x,如果x等于item,就将它的下标i添加到列表中。
- 最终,id列表中存储了所有等于set_Global_Time中元素的下标值。
相关问题
class PointnetSAModuleMSG(_PointnetSAModuleBase): """Pointnet set abstraction layer with multiscale grouping""" def __init__(self, *, npoint: int, radii: List[float], nsamples: List[int], mlps: List[List[int]], bn: bool = True, use_xyz: bool = True, pool_method='max_pool', instance_norm=False): """ :param npoint: int :param radii: list of float, list of radii to group with :param nsamples: list of int, number of samples in each ball query :param mlps: list of list of int, spec of the pointnet before the global pooling for each scale :param bn: whether to use batchnorm :param use_xyz: :param pool_method: max_pool / avg_pool :param instance_norm: whether to use instance_norm """ super().__init__() assert len(radii) == len(nsamples) == len(mlps) self.npoint = npoint self.groupers = nn.ModuleList() self.mlps = nn.ModuleList() for i in range(len(radii)): radius = radii[i] nsample = nsamples[i] self.groupers.append( pointnet2_utils.QueryAndGroup(radius, nsample, use_xyz=use_xyz) if npoint is not None else pointnet2_utils.GroupAll(use_xyz) ) mlp_spec = mlps[i] if use_xyz: mlp_spec[0] += 3 self.mlps.append(pt_utils.SharedMLP(mlp_spec, bn=bn, instance_norm=instance_norm)) self.pool_method = pool_method我想将以上模块输出的张量的shape在模块尾部打印出来方便检查,你可以提供详细的代码吗?
当模块输出的张量维度较多时,可以使用 `torch.Size` 或者 `tuple` 将它们打印出来。以下是添加打印输出的代码示例:
```
class PointnetSAModuleMSG(_PointnetSAModuleBase):
"""Pointnet set abstraction layer with multiscale grouping"""
def __init__(
self,
*,
npoint: int,
radii: List[float],
nsamples: List[int],
mlps: List[List[int]],
bn: bool = True,
use_xyz: bool = True,
pool_method='max_pool',
instance_norm=False
):
"""
:param npoint: int
:param radii: list of float, list of radii to group with
:param nsamples: list of int, number of samples in each ball query
:param mlps: list of list of int, spec of the pointnet before the global pooling for each scale
:param bn: whether to use batchnorm
:param use_xyz:
:param pool_method: max_pool / avg_pool
:param instance_norm: whether to use instance_norm
"""
super().__init__()
assert len(radii) == len(nsamples) == len(mlps)
self.npoint = npoint
self.groupers = nn.ModuleList()
self.mlps = nn.ModuleList()
for i in range(len(radii)):
radius = radii[i]
nsample = nsamples[i]
self.groupers.append(
pointnet2_utils.QueryAndGroup(radius, nsample, use_xyz=use_xyz)
if npoint is not None else pointnet2_utils.GroupAll(use_xyz)
)
mlp_spec = mlps[i]
if use_xyz:
mlp_spec[0] += 3
self.mlps.append(pt_utils.SharedMLP(mlp_spec, bn=bn, instance_norm=instance_norm))
self.pool_method = pool_method
def forward(self, xyz, features=None):
"""
:param xyz: (batch_size, num_points, 3) tensor
:param features: (batch_size, num_points, dim) tensor, optional
:return:
new_xyz: (batch_size, npoint, 3) tensor
new_features: (batch_size, npoint, \sum_k(mlps[k][-1])) tensor
"""
B, N, C = xyz.shape
if self.npoint is not None:
fps_idx = pointnet2_utils.furthest_point_sample(xyz, self.npoint)
new_xyz = pointnet2_utils.gather_operation(xyz.transpose(1, 2).contiguous(), fps_idx).transpose(1, 2).contiguous()
else:
new_xyz = None
new_features_list = []
for i, grouper in enumerate(self.groupers):
grouped_xyz, grouped_features, idx = grouper(new_xyz, xyz, features)
new_features = self.mlps[i](grouped_features)
if self.pool_method == 'max_pool':
new_features = F.max_pool1d(new_features, new_features.size(2)).squeeze(2) # (B, C, N) -> (B, C)
elif self.pool_method == 'avg_pool':
new_features = F.avg_pool1d(new_features, new_features.size(2)).squeeze(2) # (B, C, N) -> (B, C)
new_features_list.append(new_features)
new_features = torch.cat(new_features_list, dim=1)
# 打印输出张量的形状
print("new_xyz shape:", new_xyz.shape)
print("new_features shape:", new_features.shape)
return new_xyz, new_features
```
在 `forward` 方法中添加了打印输出,可以直接输出张量的形状信息。
def generate_geo_chart(data): # 统计各个地区的数量 location_counts = {} for result in data: location = result.location split_parts = split_location(location) for i, part in enumerate(split_parts): if i >= 2: # 只处理前两个元素 break if part in location_counts: location_counts[part] += 1 else: location_counts[part] = 1 # 创建 Geo 实例并设置地图类型 geo = Geo() geo.add_schema(maptype="china") # 添加数据到 Geo 实例 for part, count in location_counts.items(): try: geo.get_coordinate(part) geo.add( series_name="地域分布", data_pair=[(part, count)], type_=ChartType.EFFECT_SCATTER, # 散点图类型 symbol_size=10, # 散点大小 label_opts=opts.LabelOpts(is_show=True), # 显示标签 ) except Exception as e: print(f"坐标不存在: {part}, 错误原因: {str(e)}") # 设置全局配置项 geo.set_global_opts( visualmap_opts=opts.VisualMapOpts( max_=max(location_counts.values()), # 数据的最大值 is_piecewise=True, # 是否分段显示 ), ) # 渲染地域分布图并保存到文件 geo.width = "100%vh" geo.height = "100vh" geo.render("templates/geo_chart.html")
这段代码是一个 Python 函数,用于生成一个地域分布图。它的输入参数是一个包含数据的列表。该函数首先统计每个地区的数量,然后使用 pyecharts 库创建一个 Geo 实例,并设置地图类型为中国地图。接下来,该函数将数据添加到 Geo 实例中,以创建一个散点图。如果某些地区的坐标不存在,则该函数将显示一个错误消息。最后,该函数设置一些全局配置项,并将生成的地域分布图保存到文件中。
相关推荐
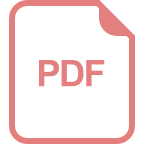
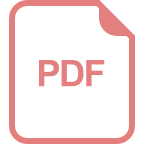












