stm32f103c8t6使用max300102的C语言程序
时间: 2023-07-30 14:07:12 浏览: 51
要使用Max300102与STM32F103C8T6进行通信,需要使用SPI接口。以下是一个简单的C语言程序,可以读取Max300102的寄存器值:
```c
#include "stm32f10x.h"
#define MAX300102_CS_PIN GPIO_Pin_4
#define MAX300102_CS_GPIO GPIOA
SPI_InitTypeDef SPI_InitStructure;
void MAX300102_SPI_init() {
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_SPI1, ENABLE);
GPIO_InitStructure.GPIO_Pin = MAX300102_CS_PIN;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(MAX300102_CS_GPIO, &GPIO_InitStructure);
GPIO_SetBits(MAX300102_CS_GPIO, MAX300102_CS_PIN);
SPI_InitStructure.SPI_Direction = SPI_Direction_2Lines_FullDuplex;
SPI_InitStructure.SPI_Mode = SPI_Mode_Master;
SPI_InitStructure.SPI_DataSize = SPI_DataSize_8b;
SPI_InitStructure.SPI_CPOL = SPI_CPOL_High;
SPI_InitStructure.SPI_CPHA = SPI_CPHA_2Edge;
SPI_InitStructure.SPI_NSS = SPI_NSS_Soft;
SPI_InitStructure.SPI_BaudRatePrescaler = SPI_BaudRatePrescaler_16;
SPI_InitStructure.SPI_FirstBit = SPI_FirstBit_MSB;
SPI_Init(SPI1, &SPI_InitStructure);
SPI_Cmd(SPI1, ENABLE);
}
uint8_t MAX300102_SPI_transfer(uint8_t data) {
while (SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_TXE) == RESET);
SPI_I2S_SendData(SPI1, data);
while (SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_RXNE) == RESET);
return SPI_I2S_ReceiveData(SPI1);
}
uint8_t MAX300102_read_register(uint8_t address) {
GPIO_ResetBits(MAX300102_CS_GPIO, MAX300102_CS_PIN);
MAX300102_SPI_transfer(0x80 | address);
uint8_t data = MAX300102_SPI_transfer(0x00);
GPIO_SetBits(MAX300102_CS_GPIO, MAX300102_CS_PIN);
return data;
}
int main(void) {
MAX300102_SPI_init();
uint8_t reg_value = MAX300102_read_register(0x01);
return 0;
}
```
这个程序初始化了SPI接口,并且提供了一个函数来读取Max300102的寄存器值。在`main()`函数中,我们使用了`MAX300102_read_register()`函数来读取地址为0x01的寄存器值,并将其存储在`reg_value`变量中。请注意,这里的CS引脚是在GPIOA的第4个引脚上,你需要根据实际情况进行修改。
相关推荐
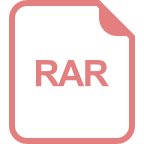
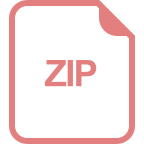
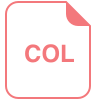
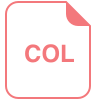
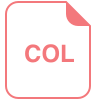
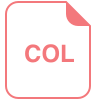
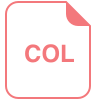









