python得到excel表中的关键词中前十权重的关键词
时间: 2023-07-07 13:28:49 浏览: 42
要实现这个任务,你可以按照以下步骤:
1.使用Python中的pandas库读取Excel表格中的数据,可以使用`pd.read_excel()`函数。
2.将每个单元格的文本内容进行分词和去停用词处理,可以使用jieba库和中文停用词表。
3.统计每个词汇在所有单元格中的出现次数,并计算每个词汇的权重。
4.按照词汇的权重进行排序,并取出前十个关键词。
以下是一份示例代码,你可以根据自己的需求进行修改和优化:
```python
import pandas as pd
import jieba
from collections import Counter
# 读取Excel表格中的数据
df = pd.read_excel('example.xlsx')
# 加载中文停用词表
stopwords = [line.strip() for line in open('stopwords.txt', 'r', encoding='utf-8').readlines()]
# 分词和去停用词处理
words = []
for cell in df.values.flatten():
if pd.isna(cell):
continue
seg_list = jieba.cut(cell)
for word in seg_list:
if word not in stopwords and len(word) > 1:
words.append(word)
# 统计词频和计算权重
word_freq = Counter(words)
total_words = sum(word_freq.values())
word_weight = {word: freq / total_words for word, freq in word_freq.items()}
# 按照权重进行排序并取出前十个关键词
top_words = sorted(word_weight.items(), key=lambda x: x[1], reverse=True)[:10]
# 输出结果
for word, weight in top_words:
print(word, weight)
```
其中,`example.xlsx`是你要处理的Excel表格文件,`stopwords.txt`是中文停用词表文件。你需要根据实际情况修改这两个文件的路径。
相关推荐
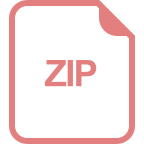
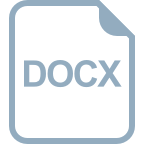
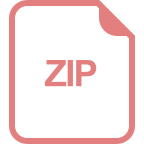
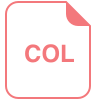
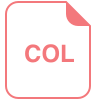
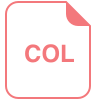
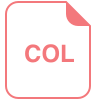
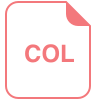









