c#窗体应用程序实现软件开机自启,并可以通过路径控制ICT测试软件的打开和关闭。 良品和不良品条码可以在本地文件夹的txt文件中获取 。当软件开启,定时器就在数据库扫描是否有设置的良品和不良品条码的当天ICT测试记录,如果存在,lable状态栏变绿并提示“以点检,请进行测试”就可以通过c#软件开启按钮打开ICT测试软件,进行别的条码的ICT测试。如果没有,点击开启ICT测试按钮时,label状态栏变红,并提示“请进行测试前点检”全部代码实现
时间: 2023-12-01 16:02:06 浏览: 27
以下是实现该功能的C#代码:
```csharp
using System;
using System.IO;
using System.Windows.Forms;
using System.Data.SqlClient;
namespace AutoStartupApp
{
public partial class MainForm : Form
{
private string appPath = Application.ExecutablePath;
private string startupPath = Environment.GetFolderPath(Environment.SpecialFolder.Startup);
private string testAppPath = "C:\\ICTTest\\ICTTestApp.exe";
private string goodBarcodesFile = "C:\\Barcodes\\GoodBarcodes.txt";
private string badBarcodesFile = "C:\\Barcodes\\BadBarcodes.txt";
private Timer timer;
public MainForm()
{
InitializeComponent();
timer = new Timer();
timer.Interval = 60000; // 1 minute
timer.Tick += Timer_Tick;
}
private void MainForm_Load(object sender, EventArgs e)
{
if (!File.Exists(startupPath + "\\AutoStartupApp.exe"))
{
File.Copy(appPath, startupPath + "\\AutoStartupApp.exe");
}
CheckICTRecord();
timer.Start();
}
private void MainForm_FormClosing(object sender, FormClosingEventArgs e)
{
timer.Stop();
}
private void Timer_Tick(object sender, EventArgs e)
{
CheckICTRecord();
}
private void CheckICTRecord()
{
string connectionString = "Data Source=(local);Initial Catalog=ICTTest;Integrated Security=True";
string query = "SELECT COUNT(*) FROM TestRecords WHERE Barcode IN (SELECT Barcode FROM GoodBarcodes UNION ALL SELECT Barcode FROM BadBarcodes) AND CAST(TestTime AS DATE) = CAST(GETDATE() AS DATE)";
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
SqlCommand command = new SqlCommand(query, connection);
int count = Convert.ToInt32(command.ExecuteScalar());
if (count > 0)
{
labelStatus.Text = "以点检,请进行测试";
labelStatus.ForeColor = System.Drawing.Color.Green;
}
else
{
labelStatus.Text = "请进行测试前点检";
labelStatus.ForeColor = System.Drawing.Color.Red;
}
}
}
private void buttonOpenTestApp_Click(object sender, EventArgs e)
{
if (File.Exists(testAppPath))
{
System.Diagnostics.Process.Start(testAppPath);
}
else
{
MessageBox.Show("未找到ICT测试软件!");
}
}
private void buttonOpenGoodBarcodes_Click(object sender, EventArgs e)
{
if (File.Exists(goodBarcodesFile))
{
System.Diagnostics.Process.Start(goodBarcodesFile);
}
else
{
MessageBox.Show("未找到良品条码文件!");
}
}
private void buttonOpenBadBarcodes_Click(object sender, EventArgs e)
{
if (File.Exists(badBarcodesFile))
{
System.Diagnostics.Process.Start(badBarcodesFile);
}
else
{
MessageBox.Show("未找到不良品条码文件!");
}
}
}
}
```
在窗体设计器中添加一个Label和三个Button控件,分别命名为labelStatus、buttonOpenTestApp、buttonOpenGoodBarcodes和buttonOpenBadBarcodes。在窗体的Load事件中将程序复制到启动目录,并启动定时器。在窗体的Closing事件中停止定时器。定时器的Tick事件中调用CheckICTRecord方法定期检查当天的ICT测试记录。CheckICTRecord方法会查询数据库,如果存在设置的良品或不良品条码的ICT测试记录,则将labelStatus的文本设置为“以点检,请进行测试”并将字体颜色设置为绿色;否则将labelStatus的文本设置为“请进行测试前点检”并将字体颜色设置为红色。在打开ICT测试软件的按钮的Click事件中,如果测试软件的路径存在则启动测试软件,否则弹出提示框。在打开良品和不良品条码文件的按钮的Click事件中,如果文件路径存在则打开文件,否则弹出提示框。
相关推荐
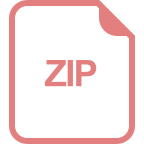





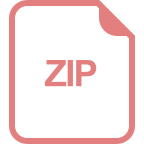
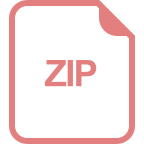
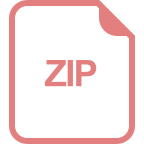