python简易购物系统现金识别支付代码
时间: 2023-09-29 22:06:40 浏览: 100
以下是一个使用OpenCV库和机器学习模型实现货币识别的Python代码示例,可供参考:
```python
import cv2
import numpy as np
import tensorflow as tf
# 加载机器学习模型
model = tf.keras.models.load_model('currency_classifier.h5')
# 货币符号和对应的值
currency_symbols = {'1': 1, '2': 2, '5': 5, '10': 10, '20': 20, '50': 50, '100': 100}
# 读取货币图像并识别金额
def recognize_currency(image):
# 转换为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 进行二值化处理
ret, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)
# 查找轮廓
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 确定图像中货币的数量
n_currencies = len(contours)
# 遍历所有货币图像
for i in range(n_currencies):
# 获取当前货币的轮廓
cnt = contours[i]
# 获取当前货币的边框
x, y, w, h = cv2.boundingRect(cnt)
# 提取当前货币的图像
currency_image = gray[y:y + h, x:x + w]
# 调整货币图像的大小
currency_image = cv2.resize(currency_image, (64, 64))
# 将货币图像转换为模型的输入格式
currency_image = np.array(currency_image).reshape(-1, 64, 64, 1) / 255.0
# 使用模型预测货币的类别
prediction = model.predict(currency_image)
# 获取货币的类别编号
currency_class = np.argmax(prediction)
# 获取货币的类别符号
currency_symbol = str(currency_class + 1)
# 获取货币的价值
currency_value = currency_symbols[currency_symbol]
# 将货币的价值添加到总金额中
total_value += currency_value
return total_value
# 主函数
if __name__ == '__main__':
# 打开摄像头
cap = cv2.VideoCapture(0)
# 循环读取图像并进行识别
while True:
# 读取图像
ret, frame = cap.read()
# 显示图像
cv2.imshow('frame', frame)
# 检测是否按下了“q”键
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 检查是否选择了现金支付
if payment_method == 'cash':
# 识别货币图像并计算总金额
total_value = recognize_currency(frame)
# 显示总金额
print('Total value:', total_value)
```
这是一个简单的示例代码,您需要根据您的具体需求进行适当的修改和优化。
阅读全文
相关推荐
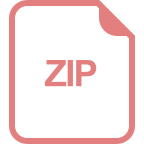
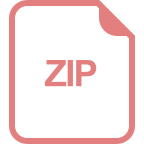
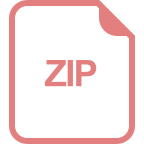
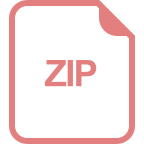
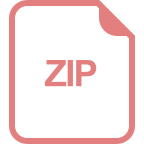
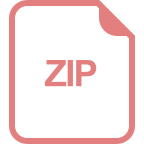
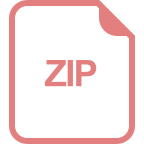
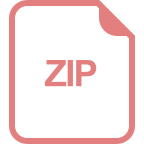
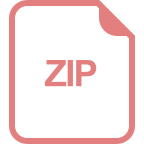
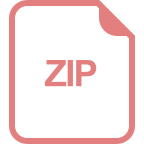
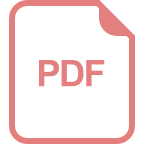