c# 通过值查询key
时间: 2024-09-28 20:04:08 浏览: 37
在 C# 中,如果你想要通过键(Key)来查询某个值,通常你会使用 Dictionary 或者 HashTable 这样的哈希集合。这两种数据结构都是基于哈希表实现的,它们提供了一个 O(1) 的平均时间复杂度来进行查找操作。
例如,如果你有一个 Dictionary<String, int>:
```csharp
Dictionary<string, int> myDict = new Dictionary<string, int>();
myDict.Add("Apple", 5);
myDict.Add("Banana", 7);
// 通过键查询值
int value;
if (myDict.TryGetValue("Apple", out value))
{
Console.WriteLine($"The value for 'Apple' is {value}");
}
```
在这个例子中,`TryGetValue` 方法尝试从字典中获取指定键对应的值。如果键存在,它会返回值并设置 `out` 参数;如果不存在,不会抛出异常,而是直接返回默认值(在这里,`out value` 将保持其原始未初始化状态)。
相关问题
c#根据值获取key
在C#中,如果需要根据值获取对应的key,可以使用Dictionary<TKey, TValue>类的LINQ扩展方法来实现。
假设有一个Dictionary<string, int>类型的字典,需要根据值获取对应的key,示例代码如下:
```csharp
Dictionary<string, int> dict = new Dictionary<string, int>()
{
{ "apple", 1 },
{ "banana", 2 },
{ "orange", 3 },
};
int value = 2;
string key = dict.FirstOrDefault(x => x.Value == value).Key;
// key = "banana"
```
以上代码中,使用FirstOrDefault方法获取第一个满足条件的元素,条件为字典中的值等于指定的value。然后获取该元素的key值,即可得到根据值获取的key。
c# Dictionary 查询key是类的值
在 C# 中,`Dictionary<TKey, TValue>` 是一种关联容器,它的键值对是泛型的,可以存储任意类型的 key 和 value。如果你想查询 key 是否是某个类的对象实例,你需要将这个类的实例作为 key 存入 Dictionary。
例如,假设你有一个名为 `Person` 的类,你可以这样做:
```csharp
public class Person
{
public string Name { get; set; }
}
// 创建一个 Dictionary,其中 Key 是 Person 类的实例
Dictionary<Person, int> personAgeDict = new Dictionary<Person, int>();
// 添加条目,比如
personAgeDict.Add(new Person() { Name = "John" }, 25);
// 现在如果你想查找特定名字的人,可以这样查询:
if (personAgeDict.ContainsKey(personToFind))
{
int age = personAgeDict[personToFind]; // 获取年龄
}
```
这里,`ContainsKey()` 方法会检查指定的 `Person` 对象是否存在作为键。如果存在,你可以通过索引 `[ ]` 访问对应的值。
阅读全文
相关推荐
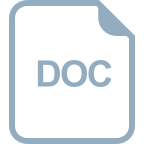
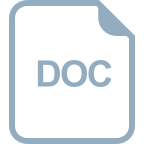
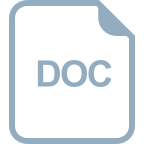











