用c++语言编写一个完整代码创建一个派生类 EBook,继承自 Book 类,并增加一些新的成员变量,如电子书的文件格式等。这个类中也可以包含构造函数、析构函数等成员函数。 在主函数中,可以创建一个指向 Book 类的指针数组,然后动态地创建 Book 类的对象和 EBook 类的对象,将这些对象存储到指针数组中。然后,可以通过循环来调用每个对象的 print() 函数,实现多态输出。
时间: 2023-02-09 17:41:49 浏览: 64
下面是示例代码,请注意,这只是一个示例,并非完整的程序,你需要根据自己的需要调整代码:
```
#include <iostream>
#include <string>
using namespace std;
// 定义 Book 类
class Book {
public:
Book(string title, string author) {
this->title = title;
this->author = author;
}
virtual void print() {
cout << "Book: " << title << ", Author: " << author << endl;
}
virtual ~Book() {}
protected:
string title;
string author;
};
// 定义 EBook 类,继承自 Book 类
class EBook: public Book {
public:
EBook(string title, string author, string format): Book(title, author) {
this->format = format;
}
void print() {
cout << "EBook: " << title << ", Author: " << author << ", Format: " << format << endl;
}
~EBook() {}
private:
string format;
};
int main() {
// 创建指向 Book 类的指针数组
Book* books[2];
// 动态地创建 Book 类的对象和 EBook 类的对象
books[0] = new Book("The Great Gatsby", "F. Scott Fitzgerald");
books[1] = new EBook("The Art of Computer Programming", "Donald Knuth", "PDF");
// 通过循环来调用每个对象的 print() 函数,实现多态输出
for (int i = 0; i < 2; i++) {
books[i]->print();
}
// 释放动态分配的内存
delete books[0];
delete books[1];
return 0;
}
```
相关推荐
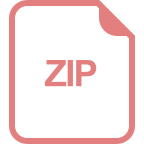
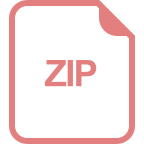




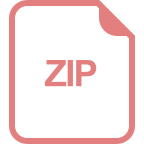
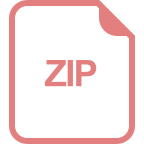
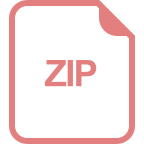
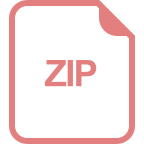
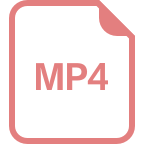
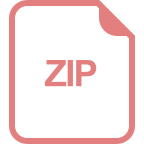