上面不算一个问题,请用Java写一个遍历目录的程序,将每天新增的文件信息存到数据库中,目录的结构为YYYY/MM
时间: 2024-02-27 12:58:58 浏览: 65
以下是一个遍历目录的Java程序,将每天新增的文件信息存储到数据库中,目录结构为YYYY/MM:
```java
import java.io.File;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class TraverseDirectory {
private static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
private static final String DB_URL = "jdbc:mysql://localhost:3306/testdb";
private static final String USER = "username";
private static final String PASS = "password";
public static void main(String[] args) {
Connection conn = null;
PreparedStatement stmt = null;
try {
Class.forName(JDBC_DRIVER);
conn = DriverManager.getConnection(DB_URL, USER, PASS);
// 设置目录路径
String path = "D:/data";
// 获取当前日期
SimpleDateFormat sdf = new SimpleDateFormat("yyyy/MM");
String currentDate = sdf.format(new Date());
// 遍历目录
File file = new File(path);
File[] fileList = file.listFiles();
for (File f : fileList) {
if (f.isDirectory() && f.getName().equals(currentDate)) {
File[] currentMonthFiles = f.listFiles();
for (File currentMonthFile : currentMonthFiles) {
String fileName = currentMonthFile.getName();
long fileSize = currentMonthFile.length();
Date fileDate = new Date(currentMonthFile.lastModified());
sdf = new SimpleDateFormat("yyyy-MM-dd");
String fileDateString = sdf.format(fileDate);
// 存储文件信息到数据库中
String sql = "INSERT INTO files (name, size, date) VALUES (?, ?, ?)";
stmt = conn.prepareStatement(sql);
stmt.setString(1, fileName);
stmt.setLong(2, fileSize);
stmt.setString(3, fileDateString);
stmt.executeUpdate();
}
}
}
} catch (ClassNotFoundException | SQLException e) {
e.printStackTrace();
} finally {
try {
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
该程序使用Java语言编写,遍历指定目录下的文件,将每天新增的文件信息存储到MySQL数据库中。程序中设定的目录路径为"D:/data",可以根据需要进行修改。另外,数据库连接信息、表名、字段名等也需要根据实际情况进行修改。
阅读全文
相关推荐
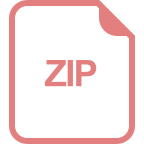
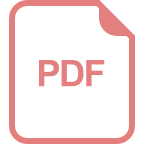
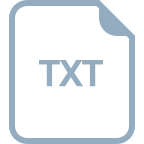
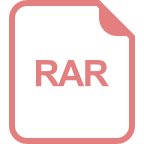
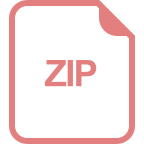
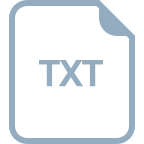
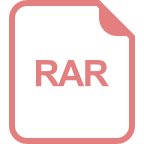
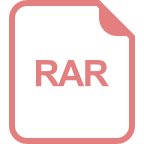
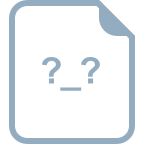
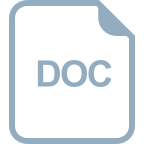
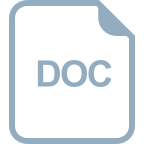
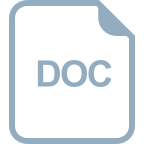
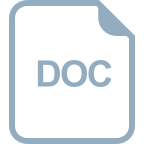
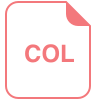
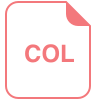
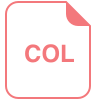