opencv c++ 水果识别
时间: 2023-09-10 15:11:39 浏览: 202
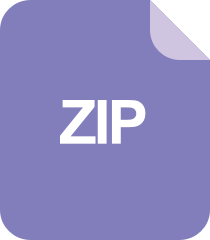
opencv水果识别样本(苹果、香蕉、梨子)
要实现基于OpenCV C++的水果识别,可以按照以下步骤进行:
1. 收集水果图片数据集,可以从网上下载或者自己拍摄。
2. 通过OpenCV读取图片,并进行预处理,如图像缩放、灰度化、滤波等。
3. 提取图像的特征,可以使用颜色直方图、纹理特征等。
4. 选择合适的分类器,如支持向量机(SVM)、朴素贝叶斯分类器(Naive Bayes)等。
5. 训练分类器,使用收集的数据集进行训练。
6. 对新的水果图片进行分类,提取特征后使用训练好的分类器进行分类。
以下是一个基于OpenCV C++和SVM的水果识别示例代码:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
using namespace cv;
int main()
{
// 读取训练数据
ifstream file("train_data.txt");
if (!file.is_open())
{
cout << "Error reading file!" << endl;
return -1;
}
Mat train_data, train_labels;
while (!file.eof())
{
string line;
getline(file, line);
if (line.empty()) continue;
vector<string> tokens;
stringstream ss(line);
string token;
while (getline(ss, token, ','))
{
tokens.push_back(token);
}
if (tokens.size() != 17) continue;
Mat row_data = Mat::zeros(1, 16, CV_32F);
for (int i = 1; i < 17; i++)
{
row_data.at<float>(i - 1) = stof(tokens[i]);
}
train_data.push_back(row_data);
train_labels.push_back(stoi(tokens[0]));
}
// 训练SVM分类器
Ptr<ml::SVM> svm = ml::SVM::create();
svm->setType(ml::SVM::C_SVC);
svm->setKernel(ml::SVM::RBF);
svm->train(train_data, ml::ROW_SAMPLE, train_labels);
// 读取测试图片
Mat img = imread("test.jpg");
if (img.empty())
{
cout << "Error reading image file!" << endl;
return -1;
}
// 预处理图像
Mat gray, blur, thresh;
cvtColor(img, gray, COLOR_BGR2GRAY);
GaussianBlur(gray, blur, Size(5, 5), 0);
threshold(blur, thresh, 0, 255, THRESH_BINARY_INV | THRESH_OTSU);
// 提取图像特征
Mat hist = Mat::zeros(1, 16, CV_32F);
int bin_width = 256 / 16;
for (int i = 0; i < thresh.rows; i++)
{
for (int j = 0; j < thresh.cols; j++)
{
int bin_idx = thresh.at<uchar>(i, j) / bin_width;
hist.at<float>(bin_idx) += 1;
}
}
normalize(hist, hist);
// 使用SVM分类器进行分类
float result = svm->predict(hist);
cout << "The fruit is: " << result << endl;
return 0;
}
```
其中,train_data.txt是训练数据集,每行数据格式为:标签,特征1,特征2,...,特征16。test.jpg是要进行分类的测试图片。在本示例中,使用颜色直方图作为图像特征,使用SVM分类器进行分类。
阅读全文
相关推荐


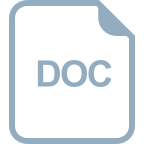

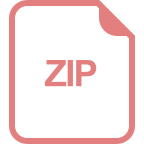
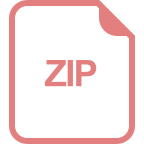
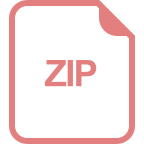
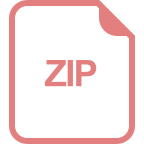
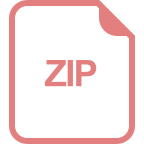
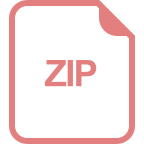
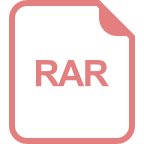
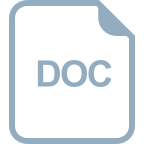
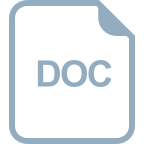
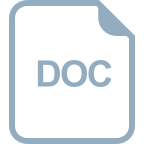
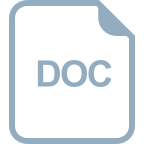
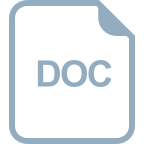