编写简单的消息发布器与订阅器,消息由浮点数和字符串两种数据类型组成,要求发布器用C++编写,订阅器用python编写
时间: 2024-01-15 21:05:48 浏览: 35
发布器(C++)代码如下:
```c++
#include <iostream>
#include <string>
#include <vector>
#include <cstring>
#include <unistd.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
using namespace std;
struct msgbuf {
long mtype;
char mtext[1024];
};
int main() {
key_t key = ftok("message_queue", 1);
int msgid = msgget(key, IPC_CREAT | 0666);
if (msgid == -1) {
cerr << "Error creating message queue" << endl;
return -1;
}
while (true) {
double num;
string str;
cout << "Enter a number or a string: ";
if (cin >> num) {
msgbuf buf;
buf.mtype = 1;
memcpy(buf.mtext, &num, sizeof(num));
msgsnd(msgid, &buf, sizeof(num), 0);
} else {
cin.clear();
cin.ignore();
getline(cin, str);
msgbuf buf;
buf.mtype = 2;
strcpy(buf.mtext, str.c_str());
msgsnd(msgid, &buf, str.size() + 1, 0);
}
}
return 0;
}
```
订阅器(python)代码如下:
```python
import struct
import sys
import os
import time
from ctypes import *
from numpy import *
class msgbuf(Structure):
_fields_ = [
("mtype", c_long),
("mtext", c_char * 1024)
]
def main():
key = os.ftok("message_queue", 1)
msgid = os.msgget(key, 0666)
if msgid == -1:
print("Error creating message queue")
return -1
while True:
buf = msgbuf()
if os.msgrcv(msgid, byref(buf), sizeof(double), 1, os.MSG_NOERROR | os.IPC_NOWAIT) != -1:
num = struct.unpack('d', buf.mtext)[0]
print(f"Received number: {num}")
if os.msgrcv(msgid, byref(buf), sizeof(buf.mtext), 2, os.MSG_NOERROR | os.IPC_NOWAIT) != -1:
str_ = buf.mtext.decode('utf-8')
print(f"Received string: {str_}")
time.sleep(0.5)
if __name__ == '__main__':
main()
```
这两个程序需要在同一个操作系统下运行。发布器程序会等待用户输入消息,如果是浮点数则发送消息类型为1的消息,如果是字符串则发送消息类型为2的消息。订阅器程序会不断接收消息队列中的消息,如果消息类型为1,则按照浮点数解析消息并输出;如果消息类型为2,则按照字符串解析消息并输出。
相关推荐
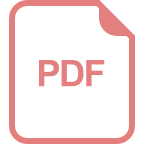
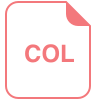
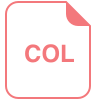
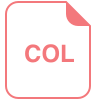
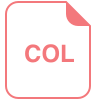
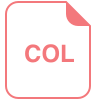









