python与C++控制流语法对比,请具体举例
时间: 2023-12-22 18:05:42 浏览: 29
好的,下面是Python和C++控制流语法的具体对比和举例:
1. 条件语句
在Python中,条件语句使用`if`关键字,例如:
```
if a > b:
print("a is greater than b")
elif a == b:
print("a is equal to b")
else:
print("a is less than b")
```
而在C++中,条件语句使用`if`和`else`关键字,例如:
```
if (a > b) {
std::cout << "a is greater than b" << std::endl;
} else if (a == b) {
std::cout << "a is equal to b" << std::endl;
} else {
std::cout << "a is less than b" << std::endl;
}
```
2. 循环语句
在Python中,循环语句使用`for`和`while`关键字,例如:
```
# for循环语句
for i in range(10):
print(i)
# while循环语句
i = 0
while i < 10:
print(i)
i += 1
```
而在C++中,循环语句使用`for`、`while`和`do-while`关键字,例如:
```
// for循环语句
for (int i = 0; i < 10; i++) {
std::cout << i << std::endl;
}
// while循环语句
int i = 0;
while (i < 10) {
std::cout << i << std::endl;
i++;
}
// do-while循环语句
int i = 0;
do {
std::cout << i << std::endl;
i++;
} while (i < 10);
```
3. 跳转语句
在Python中,跳转语句使用`break`和`continue`关键字,例如:
```
# break语句
for i in range(10):
if i == 5:
break
print(i)
# continue语句
for i in range(10):
if i == 5:
continue
print(i)
```
而在C++中,跳转语句使用`break`、`continue`和`goto`关键字,例如:
```
// break语句
for (int i = 0; i < 10; i++) {
if (i == 5) {
break;
}
std::cout << i << std::endl;
}
// continue语句
for (int i = 0; i < 10; i++) {
if (i == 5) {
continue;
}
std::cout << i << std::endl;
}
// goto语句
int i = 0;
loop:
if (i < 10) {
std::cout << i << std::endl;
i++;
goto loop;
}
```
总之,Python和C++在控制流语法上有很多不同之处,需要根据具体的需求来选择适合的编程语言。
相关推荐
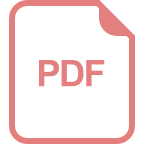
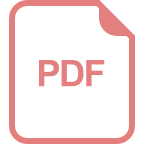
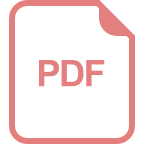














