【数据结构与算法面试指南】:循环算法专场
发布时间: 2024-09-10 10:58:13 阅读量: 217 订阅数: 77 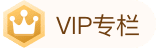
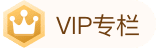
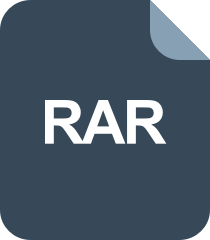
Python数据结构与经典算法讲解:深入解析与实战指南

# 1. 循环算法的基本概念和分类
在计算机科学中,循环算法是一类特殊的算法,它们通过重复执行一系列操作来解决问题。循环允许我们处理重复的任务而不需要编写冗长和重复的代码。循环算法的基本形式包括`for`循环、`while`循环以及`do-while`循环等,它们在编程语言中广泛支持,并且在不同的应用场景中发挥着核心作用。
循环算法可以按照它们的结构和用途被分类,例如:
- **线性循环**:通常用于在数组或集合中迭代元素。
- **嵌套循环**:涉及循环内再循环,用于多维数据结构或复杂逻辑的处理。
- **双重循环**:在特定问题的上下文中用于同时控制两个不同的迭代过程。
在设计循环算法时,理解循环的条件、循环体和控制变量是关键,这将有助于编写高效且易于维护的代码。在后续章节中,我们将深入探讨循环算法的理论基础和实现技巧,以及如何在多种编程语言中应用循环算法。
# 2. 经典循环算法的理论基础
## 2.1 循环算法的时间复杂度和空间复杂度
### 2.1.1 时间复杂度分析
在深入理解循环算法时,时间复杂度是一个不可或缺的概念。它描述了算法运行时间随输入数据量增长的速率,是衡量算法效率的关键指标之一。常见的时间复杂度包括常数时间(O(1))、对数时间(O(log n))、线性时间(O(n))、线性对数时间(O(n log n))、二次时间(O(n^2))等。
以线性搜索为例,其基本思想是遍历数组中的每一个元素,直到找到所需的目标值。该算法的时间复杂度分析如下:
```python
def linear_search(arr, target):
for index, value in enumerate(arr):
if value == target:
return index # 找到目标,返回索引
return -1 # 未找到目标,返回-1
```
逻辑分析:
- 在此线性搜索算法中,`for` 循环会从头到尾遍历数组 `arr`。
- 如果目标值 `target` 存在,最坏情况下需遍历整个数组,即循环执行 n 次(n 是数组的长度)。
- 因此,线性搜索的时间复杂度为 O(n)。
时间复杂度反映了算法运行时间的上界,特别是在处理大量数据时,一个低阶的时间复杂度意味着更好的性能。
### 2.1.2 空间复杂度分析
空间复杂度是指在算法执行过程中临时占用存储空间的大小,它与输入数据的规模密切相关。与时间复杂度类似,空间复杂度也是一个重要指标,用于衡量算法在执行过程中的内存消耗。
以递归算法实现的阶乘计算为例,其空间复杂度分析如下:
```python
def factorial(n):
if n <= 1:
return 1
return n * factorial(n-1)
```
逻辑分析:
- 此递归函数中,每一层递归调用都会创建一个新的活动记录(或帧),其中包含了函数的局部变量和返回地址。
- 递归调用的深度直接决定了活动记录的数量,因此,对于输入的 `n`,最多有 `n` 个活动记录。
- 因此,该递归阶乘函数的空间复杂度为 O(n),主要由递归调用栈的深度决定。
在实际应用中,要尽量减少空间复杂度,尤其是当处理大数据集时。优化存储空间的使用,可以提高算法的运行效率和可扩展性。
## 2.2 循环算法的常见类型和应用场景
### 2.2.1 线性循环
线性循环是最简单也是最常见的循环结构,其特点是在一系列数据上按照线性顺序进行遍历。线性循环常用于基本的搜索和操作任务,例如在数组中查找特定元素或遍历数组元素进行某种处理。
下面是一个遍历数组元素并打印的线性循环的例子:
```python
arr = [1, 2, 3, 4, 5]
for element in arr:
print(element)
```
逻辑分析:
- 上述代码中 `for` 循环遍历 `arr` 数组中的每个元素,并对每个元素执行打印操作。
- 这里不需要使用索引,Python 的 `for` 循环可以直接迭代可迭代对象。
- 在这个简单的例子中,线性循环使代码更简洁,同时易于理解。
### 2.2.2 嵌套循环
嵌套循环指的是一个循环内部包含另一个循环,这种结构通常用于处理多维数据结构,比如矩阵或者需要组合数据的场景。嵌套循环在算法中有着广泛的应用,如二维数组的处理、多层循环的递归算法等。
下面是一个嵌套循环在矩阵转置中的应用示例:
```python
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
transposed = [[0 for _ in range(len(matrix))] for _ in range(len(matrix[0]))]
for i in range(len(matrix)):
for j in range(len(matrix[0])):
transposed[j][i] = matrix[i][j]
print(transposed)
```
逻辑分析:
- 嵌套循环用来遍历矩阵的每一个元素,外层循环遍历行,内层循环遍历列。
- 通过转置索引,将元素按照转置后的行列关系放置在新的矩阵 `transposed` 中。
- 这里,两个嵌套的 `for` 循环分别处理行和列,实现矩阵的转置。
### 2.2.3 双重循环
双重循环是一种特殊的嵌套循环,它在很多经典算法中有着重要的作用,例如在解决某些动态规划问题时,双重循环用于构建状态转移表。双重循环的使用需要仔细考虑终止条件和循环变量的更新策略,以避免出现无限循环或效率低下的问题。
双重循环的一个典型应用场景是冒泡排序算法:
```python
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
array = [64, 34, 25, 12, 22, 11, 90]
sorted_array = bubble_sort(array)
print(sorted_array)
```
逻辑分析:
- 外层循环 `i` 控制总的遍历次数,从第一个元素到倒数第二个元素。
- 内层循环 `j` 控制在每一趟遍历中的相邻元素比较和交换,确保最大元素被放置在最后。
- 每完成一次内层循环,数组的尾部就会有一个元素被正确地排序。
## 2.3 循环控制结构的最佳实践
### 2.3.1 break和continue的使用场景
在循环控制中,`break` 和 `continue` 关键字提供了更细粒度的控制能力,允许在循环体内部进行决策,打破常规的循环流程。
- `break` 关键字用于立即退出循环,不管循环条件是否满足,都会终止循环。
- `continue` 关键字用于跳过当前循环的剩余代码,立即开始下一次循环迭代。
下面是一个 `break` 和 `continue` 在实际应用中的例子:
```python
for i in range(1, 10):
if i % 2 == 0:
continue # 跳过偶数
if i > 5:
break # 当 i 大于 5 时退出循环
print(i) # 只打印奇数且小于等于5
```
逻辑分析:
- 该循环遍历从 1 到 9 的整数。
- 当遇到偶数时,`continue` 语句被执行,跳过当前循环的剩余部分,即不打印偶数。
- 当变量 `i` 的值大于 5 时,`break` 语句被执行,循环终止,即使循环条件 `i < 10` 仍然满足。
- 结果是,只有奇数且小于等于5的数字被打印。
### 2.3.2 循环不变式和循环变量的管理
循环不变式是在循环的每次迭代中都保持为真的命题。它对于理解循环的正确性和验证程序的正确性至关重要。同时,对循环变量的管理也是编写清晰、高效循环代码的关键。
一个好的循环不变式应当具备以下三个属性:
1. 初始化:在循环开始之前,循环不变式是正确的。
2. 保持:如果在循环的某一迭代开始时不变式是正确的,并且循环体中的操作也不改变其正确性,则在该迭代结束时不变式仍为正确。
3. 终止:在循环的最后一次迭代后,不变式应该为我们提供所需的结论。
让我们以循环不变式的思想,分析以下代码段:
```python
array = [1, 2, 3, 4, 5]
sum = 0
for i in range(len(array)):
sum += array[i]
```
逻辑分析:
- 循环不变式可以设定为 `sum = array[0] + array[1] + ... + array[i-1]`,即在进入第 `i` 次迭代时,`sum` 变量已经累加了从数组开始到当前位置 `i`(不包括 `i`)的所有元素。
- 初始化:在循环开始前,空的 `sum` 变量即是数组的第一个元素之前的累积值,
0
0
相关推荐





