使用Verilog写一个4位流水灯,并再写一个对应的仿真测试文件
时间: 2024-04-08 09:29:54 浏览: 82
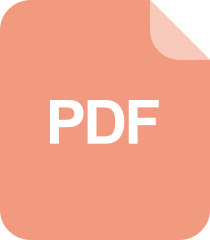
流水灯基于Verilog语言实现及测试代码.pdf
Sure! Here's an example of a 4-bit shift register implementation using Verilog:
```verilog
module shift_register (
input wire clk,
input wire reset,
output wire [3:0] led
);
reg [3:0] shift_reg;
always @(posedge clk or posedge reset) begin
if (reset)
shift_reg <= 4'b0000;
else
shift_reg <= {shift_reg[2:0], shift_reg[3]};
end
assign led = shift_reg;
endmodule
```
And here's an example of a corresponding testbench for simulation:
```verilog
module shift_register_tb;
reg clk;
reg reset;
wire [3:0] led;
shift_register dut (
.clk(clk),
.reset(reset),
.led(led)
);
always #5 clk = ~clk;
initial begin
clk = 0;
reset = 1;
#10 reset = 0;
#100 $finish;
end
endmodule
```
In this example, the `shift_register` module represents the 4-bit shift register. It has inputs for clock (`clk`) and reset (`reset`), and an output for the LED values (`led`). The shift register shifts its contents to the left on every rising edge of the clock, except when the reset signal is active.
The testbench module, `shift_register_tb`, instantiates the `shift_register` module and provides stimulus for simulation. It toggles the clock signal every 5 time units and asserts the reset signal initially for 10 time units. Finally, it finishes simulation after 100 time units.
You can use a Verilog simulator like ModelSim or EDA Playground to run this code and observe the behavior of the shift register.
阅读全文
相关推荐
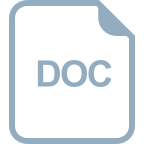
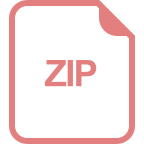
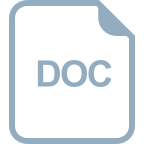
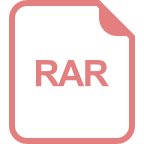
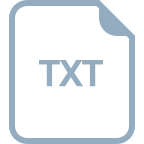
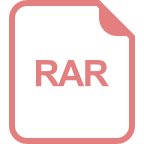
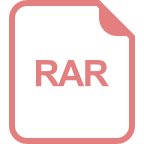
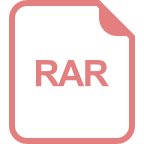
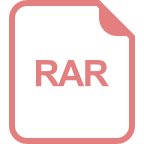
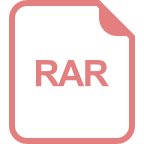
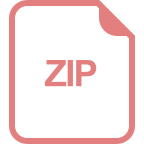
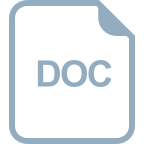
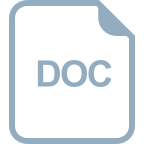
