spring boot api 调用 使用 key
时间: 2024-01-29 19:03:36 浏览: 140
如果你想要在Spring Boot应用中实现API Key鉴权,可以按照以下步骤进行操作:
1.在你的Spring Boot应用中添加Spring Security依赖,例如:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
```
2.创建一个实现`WebSecurityConfigurerAdapter`的配置类,并重写`configure(HttpSecurity http)`方法,对请求进行安全配置。例如,可以配置只有带有正确API Key的请求才能访问:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
private static final String API_KEY = "your-api-key";
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/api/**").hasAuthority("API_KEY")
.anyRequest().authenticated()
.and()
.addFilterBefore(new ApiKeyAuthenticationFilter(API_KEY), UsernamePasswordAuthenticationFilter.class);
}
}
```
3.实现一个自定义的`AuthenticationFilter`,用于API Key的验证。例如,可以实现一个`ApiKeyAuthenticationFilter`:
```java
public class ApiKeyAuthenticationFilter extends OncePerRequestFilter {
private final String apiKey;
public ApiKeyAuthenticationFilter(String apiKey) {
this.apiKey = apiKey;
}
@Override
protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain filterChain) throws ServletException, IOException {
String apiKey = request.getHeader("X-API-KEY");
if (this.apiKey.equals(apiKey)) {
UsernamePasswordAuthenticationToken authentication = new UsernamePasswordAuthenticationToken(null, null, Collections.singleton(new SimpleGrantedAuthority("API_KEY")));
SecurityContextHolder.getContext().setAuthentication(authentication);
}
filterChain.doFilter(request, response);
}
}
```
这样,就可以在Spring Boot应用中实现API Key鉴权了。当请求带有正确的API Key时,就能够通过安全认证,否则将被拒绝访问。
阅读全文
相关推荐
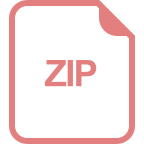
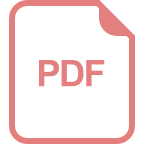
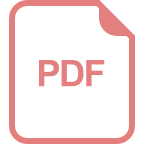
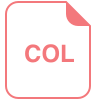
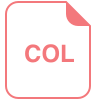
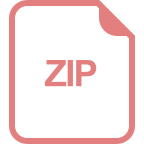
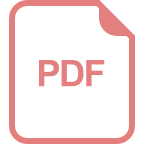
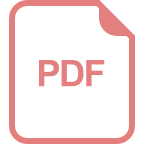
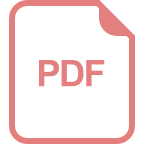
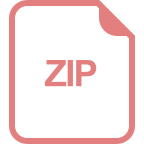
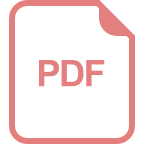
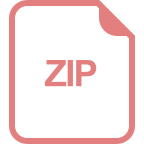
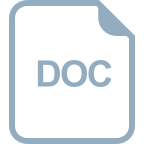
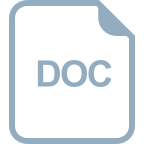
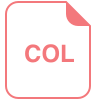
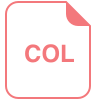

