半监督学习的随机森林分类给出分类,结果误差,可视化
时间: 2023-07-13 22:34:12 浏览: 184
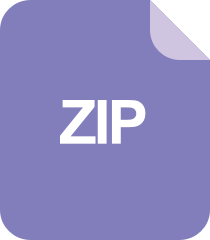
基于随机森林算法的数据分类预测
半监督学习的随机森林分类器可以用来对未标记的数据进行分类,这里给出一个示例代码,包括数据的可视化和模型分类结果的可视化。
```python
from sklearn.datasets import make_classification
from sklearn.ensemble import RandomForestClassifier
from sklearn.semi_supervised import LabelPropagation
from sklearn.metrics import accuracy_score
import matplotlib.pyplot as plt
import numpy as np
# 生成随机数据集
X, y = make_classification(n_samples=100, n_features=2, n_redundant=0,
n_classes=2, random_state=42)
# 随机选择一些样本作为有标记的数据
labeled_indices = np.random.choice(np.arange(len(y)), size=20, replace=False)
labels = np.copy(y)
labels[np.delete(np.arange(len(y)), labeled_indices)] = -1
# 可视化数据集
fig, ax = plt.subplots()
ax.scatter(X[:, 0], X[:, 1], c=labels, cmap='bwr')
ax.set_title('Labeled and Unlabeled Data')
plt.show()
# 使用标签传播算法来对未标记数据进行标记
lp_model = LabelPropagation(kernel='knn', n_neighbors=5, max_iter=1000)
lp_model.fit(X, labels)
unlabeled_predictions = lp_model.predict(X)
# 计算分类器的准确率
rf_model = RandomForestClassifier(n_estimators=10, random_state=42)
rf_model.fit(X[labeled_indices], y[labeled_indices])
labeled_predictions = rf_model.predict(X[labeled_indices])
accuracy = accuracy_score(y[labeled_indices], labeled_predictions)
print(f'Accuracy of Random Forest Classifier on labeled data: {accuracy}')
# 可视化分类结果
fig, ax = plt.subplots()
ax.scatter(X[:, 0], X[:, 1], c=unlabeled_predictions, cmap='bwr')
ax.set_title('Random Forest Classification of Unlabeled Data')
plt.show()
# 计算分类结果的误差
labeled_error = np.sum(y[labeled_indices] != labeled_predictions) / len(labeled_predictions)
unlabeled_error = np.sum(y != unlabeled_predictions) / len(unlabeled_predictions)
print(f'Labeled Error: {labeled_error}')
print(f'Unlabeled Error: {unlabeled_error}')
```
该代码首先生成一个二维随机数据集,并随机选择一些样本作为有标记的数据。然后,它使用标签传播算法来对未标记数据进行分类,并将分类结果可视化。接下来,它使用随机森林分类器对有标记的数据进行分类,并计算分类器的准确率。最后,它计算分类结果的误差,并输出结果。
您可以根据需要调整参数,例如选择不同的随机森林分类器或更改有标记数据的数量。
阅读全文
相关推荐
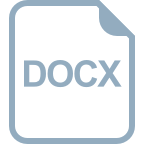

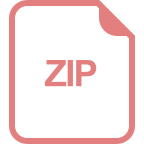
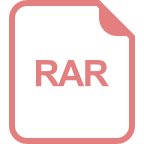
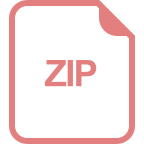
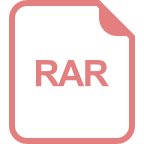
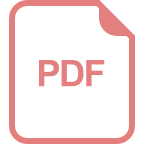
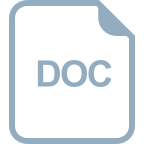
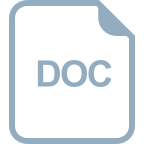
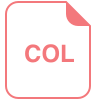
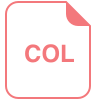
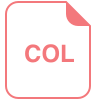
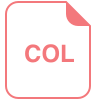
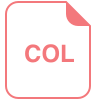
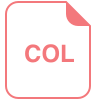
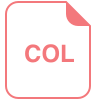
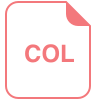
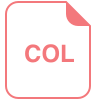