fgets
时间: 2023-08-11 22:15:00 浏览: 35
fgets() 是 C 语言中的一个标准库函数,用于从指定的文件流中读取一行字符串。它的语法如下:
```c
char* fgets(char* str, int num, FILE* stream);
```
其中,str 是一个指向 char 类型的数组,用于存储读取到的字符串;num 是要读取的最大字符数(包括空字符);stream 是一个指向 FILE 结构体的指针,表示要从哪个文件流中读取。
fgets() 函数会从文件流中读取一行字符串,直到遇到换行符('\n')或文件结束符(EOF)。它会将读取到的字符串存储到 str 中,并在字符串末尾添加一个空字符。如果读取的字符串长度超过了 num-1,那么 fgets() 只会读取前面的 num-1 个字符,剩余的字符会留在文件流中等待下一次读取。
fgets() 函数会返回一个指向 str 的指针,如果读取成功,那么这个指针就等于 str;如果读取失败,那么这个指针就等于 NULL。
相关问题
fgets和fgets_s
`fgets` 和 `fgets_s` 都是用于从文件或标准输入流中读取字符串的函数。它们的功能相似,但 `fgets_s` 是 C11 标准中引入的更加安全的版本。
`fgets` 函数的原型如下:
```c
char *fgets(char *str, int n, FILE *stream);
```
它从指定的文件流 `stream` 中读取字符,最多读取 `n - 1` 个字符的数据,并将其存储到 `str` 中。读取操作会在读取到换行符、文件结束符或达到最大字符数时停止。`fgets` 会保留读取到的换行符,并在字符串末尾添加一个 null 字符。如果成功读取,返回值是 `str` 的地址,否则返回 NULL。
`fgets_s` 函数的原型如下:
```c
errno_t fgets_s(char *str, rsize_t n, FILE *stream);
```
它与 `fgets` 的用法类似,不同之处在于:它不会保留换行符并在字符串末尾添加 null 字符;而且它还提供了更好的安全性,避免了缓冲区溢出的风险。如果成功读取,返回值为零;否则,返回一个非零值表示错误。
需要注意的是,`fgets_s` 是 C11 标准中引入的函数,在某些平台上可能不被支持。为了兼容性,可以使用条件编译来选择使用 `fgets` 或 `fgets_s`。例如:
```c
#ifdef __STDC_LIB_EXT1__
fgets_s(str, sizeof(str), stdin);
#else
fgets(str, sizeof(str), stdin);
#endif
```
这样在支持 `fgets_s` 的平台上会使用 `fgets_s`,否则会使用 `fgets`。
fgets segmentfault
segmentation fault error occurs when there is an illegal memory access in your code. It can happen for various reasons, such as dereferencing a null pointer, accessing out-of-bounds memory, or stack overflow.
In the case of "fgets," a segmentation fault can occur if you pass an invalid pointer as the first argument. Make sure that the pointer you pass to "fgets" is a valid memory address or has been properly allocated.
Here's an example of how to use "fgets" correctly:
```c
#include <stdio.h>
int main() {
char buffer[100];
FILE* file = fopen("file.txt", "r");
if (file == NULL) {
printf("File could not be opened.\n");
return 1;
}
if (fgets(buffer, sizeof(buffer), file) != NULL) {
printf("Read line: %s", buffer);
}
fclose(file);
return 0;
}
```
In this example, we open a file named "file.txt" in read mode and use "fgets" to read a line from it into the buffer. We check if the file was opened successfully and if "fgets" returns a non-null value before printing the contents of the buffer. Finally, we close the file.
Make sure to check for errors and validate your pointers when using functions that manipulate memory to avoid segmentation faults.
阅读全文
相关推荐
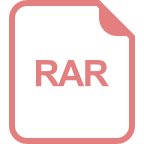










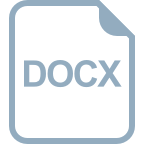
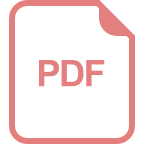
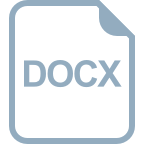