stride=2的1x1的卷积核
时间: 2024-05-23 21:16:09 浏览: 113
对于输入的特征图,使用stride=2的1x1卷积核可以实现降采样操作,即将特征图的尺寸缩小一半。这是因为1x1的卷积核只涉及通道之间的计算,而不涉及空间位置的计算。因此,对于每个位置上的像素,卷积核只会考虑该位置上每个通道的值,而不考虑周围像素的值。同时,stride=2表示卷积核每隔一个像素进行一次计算,因此输出的特征图尺寸将缩小两倍。这种操作可以用于减少特征图的尺寸,以便在后续的网络结构中提高计算效率。
相关问题
1x3和3x1卷积核代替3x3卷积核完整pytorch代码
以下是使用1x3和3x1卷积核代替3x3卷积核的PyTorch代码示例:
```python
import torch.nn as nn
# 定义一个用1x3卷积核代替3x3卷积核的卷积层
class Conv1x3(nn.Module):
def __init__(self, in_channels, out_channels):
super(Conv1x3, self).__init__()
self.conv = nn.Conv2d(in_channels, out_channels, kernel_size=(1, 3), stride=1, padding=(0, 1))
def forward(self, x):
x = self.conv(x)
return x
# 定义一个用3x1卷积核代替3x3卷积核的卷积层
class Conv3x1(nn.Module):
def __init__(self, in_channels, out_channels):
super(Conv3x1, self).__init__()
self.conv = nn.Conv2d(in_channels, out_channels, kernel_size=(3, 1), stride=1, padding=(1, 0))
def forward(self, x):
x = self.conv(x)
return x
# 定义一个包含1x3和3x1卷积层的卷积块
class ConvBlock(nn.Module):
def __init__(self, in_channels, out_channels):
super(ConvBlock, self).__init__()
self.conv1 = Conv1x3(in_channels, out_channels)
self.conv2 = Conv3x1(out_channels, out_channels)
def forward(self, x):
x = self.conv1(x)
x = self.conv2(x)
return x
```
在这个示例中,我们定义了两个卷积层,即Conv1x3和Conv3x1,分别用1x3和3x1卷积核代替3x3卷积核。然后我们定义了一个包含这两个卷积层的ConvBlock。在ConvBlock中,我们先使用Conv1x3卷积层进行卷积操作,然后再使用Conv3x1卷积层进行卷积操作。这样可以达到使用1x3和3x1卷积核代替3x3卷积核的效果。
class Net(nn.Module): def __init__(self): super(Net, self).__init__() self.conv1 = nn.Conv1d(in_channels=1, out_channels=64, kernel_size=32, stride=8, padding=12) self.pool1 = nn.MaxPool1d(kernel_size=2, stride=2) self.BN = nn.BatchNorm1d(num_features=64) self.conv3_1 = nn.Conv1d(in_channels=64, out_channels=64, kernel_size=3, stride=1, padding=1) self.pool3_1 = nn.MaxPool1d(kernel_size=2, stride=2) self.conv3_2 = nn.Conv1d(in_channels=64, out_channels=128, kernel_size=3, stride=1, padding=1) self.pool3_2 = nn.MaxPool1d(kernel_size=2, stride=2) self.conv3_3 = nn.Conv1d(in_channels=128, out_channels=256, kernel_size=3, stride=1, padding=1) self.pool3_3 = nn.MaxPool1d(kernel_size=2, stride=2) self.conv5_1 = nn.Conv1d(in_channels=64, out_channels=64, kernel_size=5, stride=1, padding=2) self.pool5_1 = nn.MaxPool1d(kernel_size=2, stride=2) self.conv5_2 = nn.Conv1d(in_channels=64, out_channels=128, kernel_size=5, stride=1, padding=2) self.pool5_2 = nn.MaxPool1d(kernel_size=2, stride=2) self.conv5_3 = nn.Conv1d(in_channels=128, out_channels=256, kernel_size=5, stride=1, padding=2) self.pool5_3 = nn.MaxPool1d(kernel_size=2, stride=2) self.conv7_1 = nn.Conv1d(in_channels=64, out_channels=64, kernel_size=7, stride=1, padding=3) self.pool7_1 = nn.MaxPool1d(kernel_size=2, stride=2) self.conv7_2 = nn.Conv1d(in_channels=64, out_channels=128, kernel_size=7, stride=1, padding=3) self.pool7_2 = nn.MaxPool1d(kernel_size=2, stride=2) self.conv7_3 = nn.Conv1d(in_channels=128, out_channels=256, kernel_size=7, stride=1, padding=3) self.pool7_3 = nn.MaxPool1d(kernel_size=2, stride=2) self.pool2 = nn.MaxPool1d(kernel_size=8, stride=1) self.fc = nn.Linear(in_features=256 * 3, out_features=4) ##这里的256*3是计算出来的 self.softmax = nn.Softmax() def forward(self, x): x = self.conv1(x) ## x:Batch, 1, 1024 x = self.pool1(x) x1 = self.conv3_1(x) x1 = self.pool3_1(x1) x1 = self.conv3_2(x1) x1 = self.pool3_2(x1) x1 = self.conv3_3(x1) x1 = self.pool3_3(x1) x2 = self.conv5_1(x) x2 = self.pool5_1(x2) x2 = self.conv5_2(x2) x2 = self.pool5_2(x2) x2 = self.conv5_3(x2) x2 = self.pool5_3(x2) x3 = self.conv7_1(x) x3 = self.pool7_1(x3) x3 = self.conv7_2(x3) x3 = self.pool7_2(x3) x3 = self.conv7_3(x3) x3 = self.pool7_3(x3) x1 = self.pool2(x1) x2 = self.pool2(x2) x3 = self.pool2(x3) Batch, Channel, Length = x1.size() x1 = x1.view(Batch, -1) Batch, Channel, Length = x2.size() x2 = x2.view(Batch, -1) Batch, Channel, Length = x3.size() x3 = x3.view(Batch, -1) x = torch.cat((x1, x2, x3), dim=1) x = self.fc(x) # x = self.softmax(x) return x,解释代码和参数,详细解释
这段代码定义了一个神经网络类,名为 Net。该类继承自 nn.Module,其初始化方法中先执行了父类的初始化。接下来定义了多个一维卷积层(Conv1d),最大池化层(MaxPool1d),以及批归一化层(BatchNorm1d)。其中每个卷积层都有输入通道数、输出通道数、卷积核大小、步幅和填充数等参数。最后还有一个全连接层(Linear),输入大小为256x3,输出大小为4。整个网络结构看起来是一个典型的卷积神经网络,用于处理一维的输入数据。
阅读全文
相关推荐
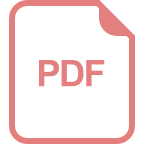
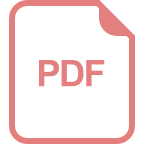
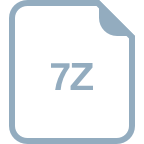














