用vue代码实现一个题库管理
时间: 2023-06-24 11:08:50 浏览: 67
好的,以下是一个简单的题库管理系统的 Vue 代码实现。
首先,我们需要安装 Vue 和 Vue Router:
```bash
npm install vue vue-router --save
```
然后,我们可以开始编写代码。首先,在 `App.vue` 中定义应用程序的基本布局和导航栏:
```html
<template>
<div id="app">
<nav>
<router-link to="/">Home</router-link>
<router-link to="/questions">Questions</router-link>
</nav>
<router-view></router-view>
</div>
</template>
<script>
export default {
name: "App",
};
</script>
<style>
nav {
background-color: #333;
color: white;
display: flex;
}
nav a {
color: white;
text-decoration: none;
margin-right: 1rem;
padding: 1rem;
}
nav a:hover {
background-color: #555;
}
</style>
```
接下来,在 `router/index.js` 中定义路由:
```js
import Vue from "vue";
import VueRouter from "vue-router";
import Home from "../views/Home.vue";
import Questions from "../views/Questions.vue";
Vue.use(VueRouter);
const routes = [
{
path: "/",
name: "home",
component: Home,
},
{
path: "/questions",
name: "questions",
component: Questions,
},
];
const router = new VueRouter({
mode: "history",
base: process.env.BASE_URL,
routes,
});
export default router;
```
然后,在 `views/Home.vue` 中定义首页:
```html
<template>
<div>
<h1>Welcome to the Question Bank Management System</h1>
<p>
This is a simple system for managing questions and answers in a database.
</p>
</div>
</template>
<script>
export default {
name: "Home",
};
</script>
<style>
h1 {
font-size: 3rem;
}
p {
font-size: 1.5rem;
}
</style>
```
最后,在 `views/Questions.vue` 中定义题库管理页面,包括一个用于显示所有问题的表格和一个用于添加新问题的表单:
```html
<template>
<div>
<h1>Question Bank</h1>
<table>
<thead>
<tr>
<th>ID</th>
<th>Question</th>
<th>Answer</th>
</tr>
</thead>
<tbody>
<tr v-for="(question, index) in questions" :key="index">
<td>{{ question.id }}</td>
<td>{{ question.question }}</td>
<td>{{ question.answer }}</td>
</tr>
</tbody>
</table>
<form @submit.prevent="addQuestion">
<label>
Question:
<input v-model="newQuestion.question" type="text" required />
</label>
<label>
Answer:
<input v-model="newQuestion.answer" type="text" required />
</label>
<button>Add</button>
</form>
</div>
</template>
<script>
export default {
name: "Questions",
data() {
return {
questions: [
{ id: 1, question: "What is 1 + 1?", answer: "2" },
{ id: 2, question: "What is the capital of France?", answer: "Paris" },
],
newQuestion: {
question: "",
answer: "",
},
};
},
methods: {
addQuestion() {
this.questions.push({
id: this.questions.length + 1,
question: this.newQuestion.question,
answer: this.newQuestion.answer,
});
this.newQuestion.question = "";
this.newQuestion.answer = "";
},
},
};
</script>
<style>
h1 {
font-size: 3rem;
}
table {
border-collapse: collapse;
margin-top: 2rem;
width: 100%;
}
th,
td {
border: 1px solid #ddd;
padding: 0.5rem;
}
th {
background-color: #f2f2f2;
}
label {
display: block;
margin-bottom: 0.5rem;
}
input[type="text"] {
width: 100%;
padding: 0.5rem;
font-size: 1rem;
border-radius: 0.25rem;
border: 1px solid #ccc;
}
button {
background-color: #4caf50;
color: white;
border: none;
border-radius: 0.25rem;
padding: 0.5rem 1rem;
margin-top: 1rem;
cursor: pointer;
}
button:hover {
background-color: #3e8e41;
}
</style>
```
这样,我们就完成了一个简单的题库管理系统。当我们在浏览器中打开应用程序并导航到 `/questions` 时,我们将看到一个表格,其中包含两个预定义的问题。我们还可以使用表单添加新的问题。
相关推荐
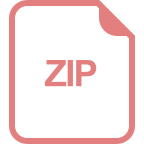














