java后端httppost提交文件流 及服务端接收文件流
时间: 2023-05-04 16:02:15 浏览: 96
Java后端可以使用HttpURLConnection类来实现HTTP请求。通过设置请求方法为POST,然后设置Content-Type为multipart/form-data,可以提交文件流到服务端。下面是代码示例:
```
URL url = new URL("http://example.com/upload");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setDoOutput(true);
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "multipart/form-data; boundary=" + boundary);
OutputStream outputStream = conn.getOutputStream();
Writer writer = new PrintWriter(new OutputStreamWriter(outputStream, charset), true);
String fileFieldName = "file";
File fileToUpload = new File("path/to/file");
writer.append("--" + boundary).append(CRLF);
writer.append("Content-Disposition: form-data; name=\"" + fileFieldName + "\"; filename=\"" + fileToUpload.getName() + "\"").append(CRLF);
writer.append("Content-Type: " + URLConnection.guessContentTypeFromName(fileToUpload.getName())).append(CRLF);
writer.append("Content-Transfer-Encoding: binary").append(CRLF);
writer.append(CRLF);
Files.copy(fileToUpload.toPath(), outputStream);
writer.append(CRLF).flush();
writer.append("--" + boundary + "--").append(CRLF).flush();
int statusCode = conn.getResponseCode();
```
服务端可以使用Java Servlet来接收文件流。通过解析HTTP请求中的Content-Type和Content-Disposition头部信息,可以得到发送的文件的信息,然后通过IO流保存上传的文件。下面是代码示例:
```
Part filePart = request.getPart("file");
InputStream fileContent = filePart.getInputStream();
String fileName = extractFileName(filePart.getHeader("Content-Disposition"));
File fileToSave = new File(uploadFilePath + File.separator + fileName);
Files.copy(fileContent, fileToSave.toPath());
```
其中,extractFileName方法可以解析Content-Disposition头部信息中的文件名:
```
private String extractFileName(String header) {
String[] parts = header.split(";");
for (String part : parts) {
if (part.trim().startsWith("filename")) {
String fileName = part.substring(part.indexOf('=') + 1).trim().replace("\"", "");
return fileName.substring(fileName.lastIndexOf('/') + 1).substring(fileName.lastIndexOf('\\') + 1);
}
}
return null;
}
```
相关推荐
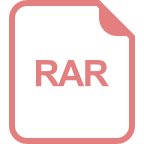
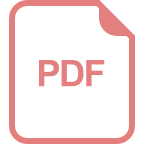
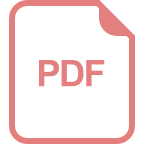













