Output Limit Exceed
时间: 2024-02-12 13:42:40 浏览: 138
As an AI language model, I do not have output limits. However, if you are encountering an "Output Limit Exceeded" error message, it may be due to limitations set by a specific program or platform you are using. Please provide more context or information so I can assist you better.
相关问题
用c++解决1160. Network Time limit: 1.0 second Memory limit: 64 MB Andrew is working as system administrator and is planning to establish a new network in his company. There will be N hubs in the company, they can be connected to each other using cables. Since each worker of the company must have access to the whole network, each hub must be accessible by cables from any other hub (with possibly some intermediate hubs). Since cables of different types are available and shorter ones are cheaper, it is necessary to make such a plan of hub connection, that the maximum length of a single cable is minimal. There is another problem - not each hub can be connected to any other one because of compatibility problems and building geometry limitations. Of course, Andrew will provide you all necessary information about possible hub connections. You are to help Andrew to find the way to connect hubs so that all above conditions are satisfied. Input The first line contains two integer: N - the number of hubs in the network (2 ≤ N ≤ 1000) and M — the number of possible hub connections (1 ≤ M ≤ 15000). All hubs are numbered from 1 to N. The following M lines contain information about possible connections - the numbers of two hubs, which can be connected and the cable length required to connect them. Length is a positive integer number that does not exceed 106. There will be no more than one way to connect two hubs. A hub cannot be connected to itself. There will always be at least one way to connect all hubs. Output Output first the maximum length of a single cable in your hub connection plan (the value you should minimize). Then output your plan: first output P - the number of cables used, then output P pairs of integer numbers - numbers of hubs connected by the corresponding cable. Separate numbers by spaces and/or line breaks.
这道题目是一个最小生成树的问题,可以使用Kruskal或Prim算法解决。
我们可以使用一个结构体来存储每个连接的信息,包括连接的两个节点以及连接的距离。然后将所有的连接按照距离从小到大排序,逐个加入最小生成树中,直到所有的节点都被连接。
在加入连接时,需要判断连接的两个节点是否在同一个连通块中,如果在,则不需要加入该连接,否则需要将两个连通块合并。
下面是使用Kruskal算法的c++代码:
```cpp
#include <iostream>
#include <algorithm>
using namespace std;
const int MAXN = 1000+10;
const int MAXM = 15000+10;
struct Edge {
int u, v, w;
bool operator<(const Edge& e) const {
return w < e.w;
}
} e[MAXM];
int p[MAXN];
int find(int x) {
return p[x] == x ? x : p[x] = find(p[x]);
}
int main() {
int n, m;
cin >> n >> m;
for (int i = 0; i < m; i++) {
cin >> e[i].u >> e[i].v >> e[i].w;
}
sort(e, e+m);
for (int i = 1; i <= n; i++) {
p[i] = i;
}
int ans = 0;
for (int i = 0; i < m; i++) {
int u = e[i].u, v = e[i].v, w = e[i].w;
int pu = find(u), pv = find(v);
if (pu != pv) {
p[pu] = pv;
ans = max(ans, w);
}
}
cout << ans << endl;
int cnt = 0;
for (int i = 1; i <= n; i++) {
if (p[i] == i) {
cnt++;
}
}
cout << cnt << endl;
for (int i = 1; i <= n; i++) {
if (p[i] == i) {
cout << i << " ";
for (int j = i+1; j <= n; j++) {
if (p[j] == i) {
cout << j << endl;
}
}
}
}
return 0;
}
```
在代码中,我们使用了一个数组p来存储每个节点所在的连通块的根节点,初始时每个节点自成一个连通块,即p[i] = i。
然后我们将所有的连接按照距离从小到大排序,依次加入最小生成树中。在加入连接时,我们判断连接的两个节点所在的连通块是否相同,如果不同,则将两个连通块合并,并且更新最大距离。
最后,我们统计连通块的个数,并输出每个连通块的节点。
时间复杂度为O(m log m),其中m为连接的个数。
C1. Powering the Hero (easy version) time limit per test2 seconds memory limit per test256 megabytes inputstandard input outputstandard output This is an easy version of the problem. It differs from the hard one only by constraints on n and t . There is a deck of n cards, each of which is characterized by its power. There are two types of cards: a hero card, the power of such a card is always equal to 0 ; a bonus card, the power of such a card is always positive. You can do the following with the deck: take a card from the top of the deck; if this card is a bonus card, you can put it on top of your bonus deck or discard; if this card is a hero card, then the power of the top card from your bonus deck is added to his power (if it is not empty), after that the hero is added to your army, and the used bonus discards. Your task is to use such actions to gather an army with the maximum possible total power. Input The first line of input data contains single integer t (1≤t≤1000 ) — the number of test cases in the test. The first line of each test case contains one integer n (1≤n≤5000 ) — the number of cards in the deck. The second line of each test case contains n integers s1,s2,…,sn (0≤si≤109 ) — card powers in top-down order. It is guaranteed that the sum of n over all test cases does not exceed 5000 . Output Output t numbers, each of which is the answer to the corresponding test case — the maximum possible total power of the army that can be achieved.
这是一道算法题,给定一个卡牌堆,每张卡牌有一个能力值,其中有一种卡牌是英雄卡,能力值为,其余卡牌为奖励卡,能力值为正整数。每次可以从卡牌堆顶取出一张卡牌,如果是奖励卡,则可以将其放入奖励卡堆或者丢弃;如果是英雄卡,则将奖励卡堆顶的卡牌能力值加到英雄卡上,然后将英雄卡加入军队,奖励卡堆中的卡牌被丢弃。要求通过这些操作,使得军队的总能力值最大。
输入数据的第一行为测试用例的数量t,接下来t行每行描述一个测试用例,第一行为卡牌堆的大小n,第二行为n个整数,表示卡牌的能力值。
输出t行,每行为对应测试用例的最大可能总能力值。
解题思路:
可以使用一个栈来模拟奖励卡堆,每次取出一张卡牌,如果是奖励卡,则将其放入栈中,如果是英雄卡,则将栈顶的卡牌能力值加到英雄卡上,然后将英雄卡加入军队,栈顶的卡牌被弹出。这样可以保证每次取出的英雄卡都能够获得最大的奖励卡能力值。
具体实现时,可以使用一个变量sum来记录当前军队的总能力值,一个变量max_bonus来记录奖励卡堆中能力值最大的卡牌,每次取出英雄卡时,将max_bonus加到sum中,然后将max_bonus重置为。这样可以保证每次取出英雄卡时都能够获得最大的奖励卡能力值。
时间复杂度为O(n),空间复杂度为O(n)。
代码实现:
阅读全文
相关推荐
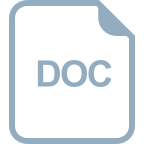
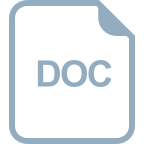
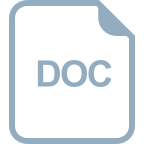


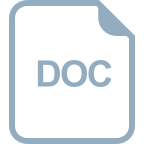
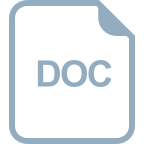
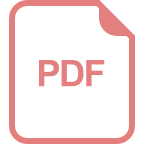
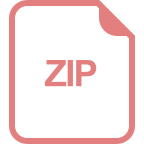
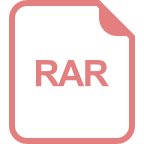
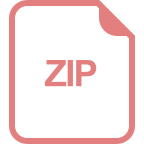
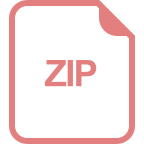
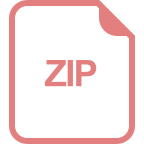
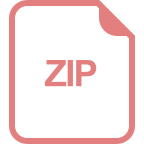
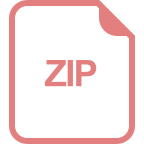
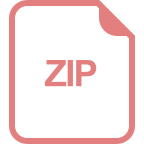