读取txt文档的三维数组C++
时间: 2023-08-15 18:08:38 浏览: 100
读取txt文档的三维数组可以通过以下步骤实现:
1. 打开txt文件,读取其中的数据并存储到一个字符串中。
2. 将字符串按照空格或换行符进行分割,得到每个数据的值。
3. 将得到的数据按照指定的行列和深度进行存储,构成一个三维数组。
以下是一段示例代码:
```c++
#include <iostream>
#include <fstream>
#include <sstream>
#include <vector>
using namespace std;
int main()
{
ifstream file("data.txt");
if(!file)
{
cout << "Failed to open file." << endl;
return 0;
}
// 读取文件内容
stringstream buffer;
buffer << file.rdbuf();
string contents = buffer.str();
// 将字符串按照空格或换行符进行分割
vector<string> values;
stringstream ss(contents);
string value;
while(ss >> value)
{
values.push_back(value);
}
// 构造三维数组
int rows = 3; // 行数
int cols = 4; // 列数
int depth = 2; // 深度
int index = 0;
int arr[rows][cols][depth];
for(int k = 0; k < depth; ++k)
{
for(int i = 0; i < rows; ++i)
{
for(int j = 0; j < cols; ++j)
{
arr[i][j][k] = stoi(values[index]);
++index;
}
}
}
// 输出结果
for(int k = 0; k < depth; ++k)
{
cout << "Depth " << k << ":" << endl;
for(int i = 0; i < rows; ++i)
{
for(int j = 0; j < cols; ++j)
{
cout << arr[i][j][k] << " ";
}
cout << endl;
}
cout << endl;
}
return 0;
}
```
其中,data.txt文件内容如下:
```
1 2 3 4
5 6 7 8
9 10 11 12
13 14 15 16
17 18 19 20
21 22 23 24
```
程序输出为:
```
Depth 0:
1 2 3 4
5 6 7 8
9 10 11 12
Depth 1:
13 14 15 16
17 18 19 20
21 22 23 24
```
相关推荐
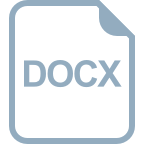
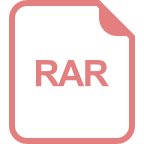
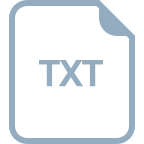














