我在Android布局文件中添加了进度条、上下曲暂停播放按钮、时间、listview组件,请帮我补全java代码
时间: 2023-11-12 20:06:06 浏览: 38
好的,以下是可能的代码实现:
```
public class MainActivity extends AppCompatActivity {
private ProgressBar progressBar;
private ImageButton playBtn, pauseBtn, prevBtn, nextBtn;
private TextView timeTextView;
private ListView listView;
private MediaPlayer mediaPlayer;
private ArrayList<String> songsList = new ArrayList<String>();
private ArrayAdapter<String> adapter;
private int currentSongIndex = 0;
private Handler mHandler = new Handler();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// 初始化各个组件
progressBar = findViewById(R.id.progress_bar);
playBtn = findViewById(R.id.play_button);
pauseBtn = findViewById(R.id.pause_button);
prevBtn = findViewById(R.id.prev_button);
nextBtn = findViewById(R.id.next_button);
timeTextView = findViewById(R.id.time_text_view);
listView = findViewById(R.id.list_view);
mediaPlayer = new MediaPlayer();
// 添加音乐
songsList.add("song1.mp3");
songsList.add("song2.mp3");
// ...
// 初始化listView的adapter
adapter = new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, songsList);
listView.setAdapter(adapter);
// 设置监听器
playBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
playSong(currentSongIndex);
}
});
pauseBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
pauseSong();
}
});
prevBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
playPrevSong();
}
});
nextBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
playNextSong();
}
});
// 设置mediaPlayer的监听器
mediaPlayer.setOnCompletionListener(new MediaPlayer.OnCompletionListener() {
@Override
public void onCompletion(MediaPlayer mediaPlayer) {
// 播放完毕后自动播放下一首
playNextSong();
}
});
}
// 播放指定位置的歌曲
private void playSong(int songIndex) {
try {
mediaPlayer.reset();
mediaPlayer.setDataSource(songsList.get(songIndex));
mediaPlayer.prepare();
mediaPlayer.start();
// 更新进度条和时间
updateProgressBar();
updateSongTime();
// 更新播放/暂停按钮的图标
playBtn.setVisibility(View.GONE);
pauseBtn.setVisibility(View.VISIBLE);
} catch (IOException e) {
e.printStackTrace();
}
}
// 暂停当前歌曲
private void pauseSong() {
mediaPlayer.pause();
// 更新播放/暂停按钮的图标
playBtn.setVisibility(View.VISIBLE);
pauseBtn.setVisibility(View.GONE);
}
// 播放上一首歌曲
private void playPrevSong() {
currentSongIndex--;
if (currentSongIndex < 0) {
currentSongIndex = songsList.size() - 1;
}
playSong(currentSongIndex);
}
// 播放下一首歌曲
private void playNextSong() {
currentSongIndex++;
if (currentSongIndex >= songsList.size()) {
currentSongIndex = 0;
}
playSong(currentSongIndex);
}
// 更新进度条和时间
private void updateProgressBar() {
mHandler.postDelayed(mUpdateTimeTask, 100);
}
private Runnable mUpdateTimeTask = new Runnable() {
public void run() {
long totalDuration = mediaPlayer.getDuration();
long currentDuration = mediaPlayer.getCurrentPosition();
// 更新进度条
int progress = (int)(getProgressPercentage(currentDuration, totalDuration));
progressBar.setProgress(progress);
// 更新时间
timeTextView.setText(milliSecondsToTimer(currentDuration) + "/" + milliSecondsToTimer(totalDuration));
// Running this thread after 100 milliseconds
mHandler.postDelayed(this, 100);
}
};
// 根据当前时间和总时间计算进度条的百分比
private int getProgressPercentage(long currentDuration, long totalDuration){
Double percentage = (double) 0;
long currentSeconds = (int) (currentDuration / 1000);
long totalSeconds = (int) (totalDuration / 1000);
// calculating percentage
percentage =(((double)currentSeconds)/totalSeconds)*100;
// return percentage
return percentage.intValue();
}
// 将毫秒转换为“00:00”格式的时间
private String milliSecondsToTimer(long milliseconds){
String finalTimerString = "";
String secondsString = "";
// Convert total duration into time
int hours = (int)( milliseconds / (1000*60*60));
int minutes = (int)(milliseconds % (1000*60*60)) / (1000*60);
int seconds = (int) ((milliseconds % (1000*60*60)) % (1000*60) / 1000);
// Add hours if there
if(hours > 0){
finalTimerString = hours + ":";
}
// Prepending 0 to seconds if it is one digit
if(seconds < 10){
secondsString = "0" + seconds;
}else{
secondsString = "" + seconds;}
finalTimerString = finalTimerString + minutes + ":" + secondsString;
// return timer string
return finalTimerString;
}
}
```
注意,上面的代码仅供参考,可能需要根据具体的需求做出修改。例如,添加音乐文件时需要将文件放在正确的位置,并且需要添加相应的权限;listView的adapter可以使用自定义的adapter等。
相关推荐
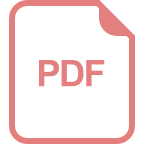
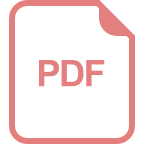














