在开发JAvaweb中基于servlet和jsp封装一套用于文件上传的方法
时间: 2024-03-08 15:50:20 浏览: 87
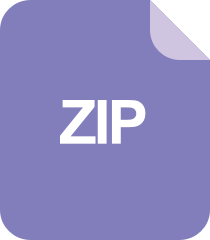
FileUploadServlet_javaweb文件上传servlet_
好的,基于Servlet和JSP实现文件上传,可以封装以下方法:
```java
public class FileUtils {
/**
* 上传文件
* @param request HttpServletRequest对象
* @param fileInputName 表单中文件上传控件的name属性值
* @param destPath 文件上传后保存的路径
* @return 上传成功返回true,否则返回false
* @throws IOException
*/
public static boolean uploadFile(HttpServletRequest request, String fileInputName, String destPath) throws IOException, ServletException {
// 获取文件上传控件
Part part = request.getPart(fileInputName);
// 获取上传文件名
String fileName = getFileName(part);
if (StringUtils.isBlank(fileName)) {
return false;
}
// 获取上传文件的后缀名
String fileSuffix = getFileSuffix(fileName);
// 生成保存文件的路径
String savePath = destPath + File.separator + UUID.randomUUID().toString() + "." + fileSuffix;
// 写入上传文件
InputStream input = part.getInputStream();
FileOutputStream output = new FileOutputStream(savePath);
int len = 0;
byte[] buffer = new byte[1024];
while ((len = input.read(buffer)) != -1) {
output.write(buffer, 0, len);
}
output.flush();
output.close();
input.close();
return true;
}
/**
* 获取上传文件名
* @param part
* @return
*/
private static String getFileName(Part part) {
String contentDisposition = part.getHeader("content-disposition");
String[] items = contentDisposition.split(";");
for (String item : items) {
if (item.trim().startsWith("filename")) {
return item.substring(item.indexOf("=") + 2, item.length() - 1);
}
}
return null;
}
/**
* 获取文件名的后缀
* @param fileName 文件名
* @return 文件后缀
*/
public static String getFileSuffix(String fileName) {
return fileName.substring(fileName.lastIndexOf(".") + 1);
}
}
```
其中,`uploadFile`方法用于上传文件,`getFileName`方法用于从上传控件获取上传的文件名,`getFileSuffix`方法用于获取文件名的后缀。需要注意的是,这里使用了`Part`类来处理文件上传,需要在Servlet 3.0及以上版本中使用。
阅读全文
相关推荐
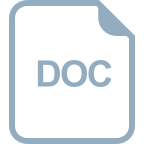
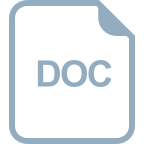
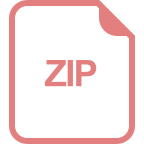
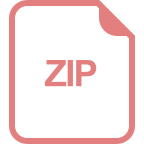
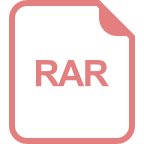
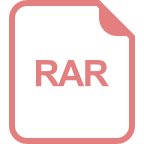
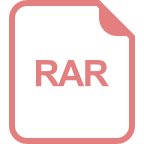
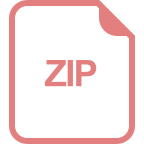
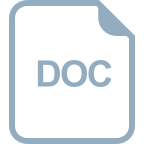
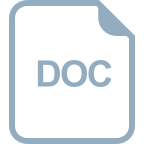
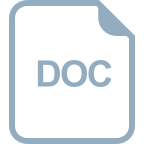
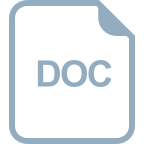
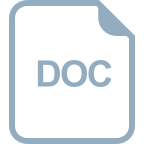
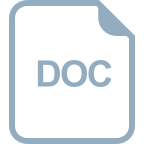

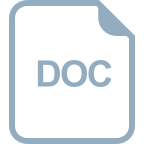
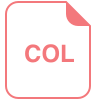
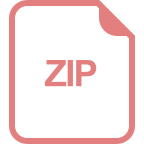