数据结构算术表达式求值具体实现代码
时间: 2023-10-17 13:13:13 浏览: 39
以下是一个简单的数据结构算术表达式求值的具体实现代码,仅供参考:
```
#include <iostream>
#include <stack>
#include <string>
#include <vector>
using namespace std;
// 定义运算符优先级
int priority(char ch) {
if (ch == '^') {
return 3;
} else if (ch == '*' || ch == '/') {
return 2;
} else if (ch == '+' || ch == '-') {
return 1;
} else {
return 0;
}
}
// 将中缀表达式转换为后缀表达式
vector<string> infix2postfix(string str) {
stack<char> opStack;
vector<string> result;
string temp;
int length = str.length();
for (int i = 0; i < length; i++) {
char ch = str[i];
if (isdigit(ch)) {
temp += ch;
} else if (ch == '.') {
temp += ch;
} else if (isalpha(ch)) {
temp += ch;
} else if (ch == '(') {
opStack.push(ch);
} else if (ch == ')') {
while (!opStack.empty() && opStack.top() != '(') {
temp = opStack.top();
result.push_back(temp);
opStack.pop();
}
if (!opStack.empty() && opStack.top() == '(') {
opStack.pop();
}
} else if (ch == '+' || ch == '-' || ch == '*' || ch == '/' || ch == '^') {
while (!opStack.empty() && opStack.top() != '(' && priority(ch) <= priority(opStack.top())) {
temp = opStack.top();
result.push_back(temp);
opStack.pop();
}
opStack.push(ch);
}
}
while (!opStack.empty()) {
temp = opStack.top();
result.push_back(temp);
opStack.pop();
}
return result;
}
// 从后缀表达式中计算结果
float evaluatePostfix(vector<string> postfix) {
stack<float> stack;
int length = postfix.size();
for (int i = 0; i < length; i++) {
string token = postfix[i];
if (isdigit(token[0])) {
float number = stof(token);
stack.push(number);
} else if (token == "+" || token == "-" || token == "*" || token == "/" || token == "^") {
float operand2 = stack.top();
stack.pop();
float operand1 = stack.top();
stack.pop();
float result = 0;
if (token == "+") {
result = operand1 + operand2;
} else if (token == "-") {
result = operand1 - operand2;
} else if (token == "*") {
result = operand1 * operand2;
} else if (token == "/") {
result = operand1 / operand2;
} else if (token == "^") {
result = pow(operand1, operand2);
}
stack.push(result);
}
}
return stack.top();
}
int main() {
string str;
cout << "请输入一个中缀表达式:";
cin >> str;
vector<string> postfix = infix2postfix(str);
float result = evaluatePostfix(postfix);
cout << "计算结果为:" << result << endl;
return 0;
}
```
相关推荐
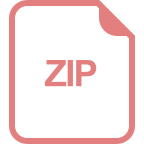
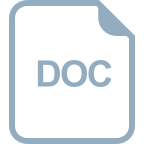
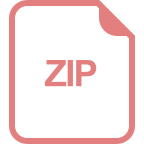









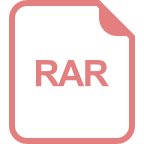
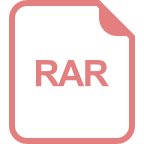
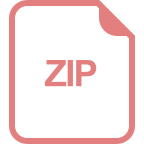
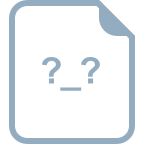
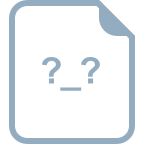
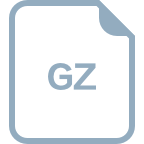