【问题描述】应用Horspool算法在一个长度为n(n<=1000)的文本中,查找一个长度为m(n>=m)的模式。如果模式位于文本串中,返回模式每次出现的位序;如果模式不在文本串中,打印输出false。文本字符均为可显示的ASCII码。
时间: 2024-03-24 14:35:47 浏览: 15
好的,这是一个字符串匹配的问题,可以使用 Horspool 算法来解决。下面是具体的实现步骤:
1. 构建坏字符表(bad table)
在 Horspool 算法中,我们需要构建一个坏字符表,用于在匹配过程中跳过尽可能多的字符。坏字符表的构建方法是,将模式串中每个字符在模式串中最右边的位置记录在表中,如果坏字符不在模式串中,则记录为 -1。
2. 匹配过程
从文本串的第一个字符开始,将模式串从右往左地与文本串匹配。如果匹配成功,返回模式串在文本串中的位置;否则,根据坏字符表跳过尽可能多的字符,继续匹配。
3. 完整代码实现
下面是使用 Python 实现的 Horspool 算法代码:
```python
def build_bad_table(pattern):
bad_table = [-1] * 256
for i in range(len(pattern)):
bad_table[ord(pattern[i])] = i
return bad_table
def horspool(text, pattern):
n, m = len(text), len(pattern)
if n < m:
return False
bad_table = build_bad_table(pattern)
i = m - 1
while i < n:
j = m - 1
while text[i] == pattern[j]:
if j == 0:
return i
i -= 1
j -= 1
i += m - min(j, 1+bad_table[ord(text[i])])
return False
text = "hello world"
pattern = "world"
pos = horspool(text, pattern)
if pos:
print("Pattern found at position", pos)
else:
print("Pattern not found")
```
注意,该算法的时间复杂度为 O(n+m),其中 n 和 m 分别为文本串和模式串的长度。
相关推荐
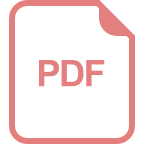
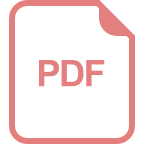







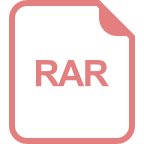
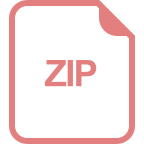
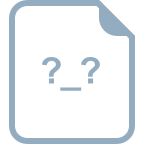
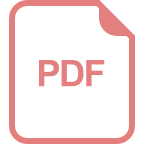
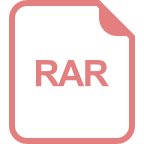